The JavaScript code snippet helps you to move focus with the keyboard arrow keys. It enables users to navigate through a table using arrow keys, specifically left, right, up, and down arrows. This code improves accessibility and ease of use for individuals who rely on keyboard navigation.
You can enhance keyboard navigation on web applications. It’s designed to be programmatically integrated into your projects that involve tabindex attribute, tables, or grids. By doing so, you can significantly improve the user experience, especially for those who rely on keyboard navigation or have mobility challenges.
How to Move Focus with Arrow Keys using JavaScript
1. First, create an HTML structure that includes your table. Ensure that each table cell (td) where you want to enable keyboard navigation has a "tabindex"
attribute. Here’s an example:
<a href="#" tabindex="1">Start</a> <p>Tab into first cell in table.</p> <p>Then, use arrow keys to change focus on cell.</p> <p>Once you're in a cell you can tab to focus on the links inside.</p> <p>Then, you can tab out of the cell and resume navigating with your arrows.</p> <table id="myTable"> <tr id="l01"> <td id="l01_1" tabindex="1"><a href="#">one</a>-<a href="#">x</a></td> <td id="l01_2" tabindex="0">two-<a href="#">x</a></td> <td id="l01_3" tabindex="0">three</td> </tr> <tr id="l02"> <td id="l02_1" tabindex="0">one -<a href="#">x</a></td> <td id="l02_2" tabindex="0"><a href="#">two</a>-<a href="#">x</td> <td id="l02_3" tabindex="0"><a href="#">three</a>-<a href="#">x</td> </tr> <tr id="l03"> <td id="l03_1" tabindex="0">one -<a href="#">x</a></td> <td id="l03_2" tabindex="0"><a href="#">two</a>-<a href="#">x</td> <td id="l03_3" tabindex="0"><a href="#">three</a>-<a href="#">x</td> </tr> <tr id="l04"> <td id="l04_1" tabindex="0">one -<a href="#">x</a></td> <td id="l04_2" tabindex="0"><a href="#">two</a>-<a href="#">x</td> <td id="l04_3" tabindex="0"><a href="#">three</a>-<a href="#">x</td> </tr> </table>
2. You can apply custom CSS to style your table and cells. (Optional)
body { font-family: "Arial"; } body table td { padding: 10px; background: pink; width: 100px; }
3. Copy and paste the following JavaScript code into a <script>
tag within your HTML document, preferably just before the closing </body>
tag. It listens for arrow key events (left, right, up, and down) within the table. When an arrow key is pressed, the code shifts the focus to the neighboring cell in the corresponding direction.
var myTable = document.getElementById('myTable'); myTable.onkeydown = function(event) { var numberOfCells = document.getElementById('myTable').getElementsByTagName("td").length; if(event.keyCode == 37) { console.log('left'); document.getElementById(event.target.id).blur() var currentfocus = event.target.id.split(''); currentfocus.splice(currentfocus.length-1, 1, +currentfocus[currentfocus.length - 1]-1); var newfocus = currentfocus.join(''); document.getElementById(newfocus).focus() } //////////// else if (event.keyCode == 39) { console.log('right'); document.getElementById(event.target.id).blur() var currentfocus = event.target.id.split(''); currentfocus.splice(currentfocus.length-1, 1, +currentfocus[currentfocus.length - 1]+1); var newfocus = currentfocus.join(''); document.getElementById(newfocus).focus() } //////////// else if (event.keyCode == 38) { console.log('up'); document.getElementById(event.target.id).blur() var currentfocus = event.target.id.split(''); currentfocus.splice(2, 1, +currentfocus[2]-1); var newfocus = currentfocus.join(''); document.getElementById(newfocus).focus(); } else if (event.keyCode == 40) { console.log('down'); document.getElementById(event.target.id).blur() var currentfocus = event.target.id.split(''); currentfocus.splice(2,1, +currentfocus[2]+1); var newfocus = currentfocus.join(''); document.getElementById(newfocus).focus(); } };
That’s all! hopefully, you have successfully created a functionality to move focus with arrow keys. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
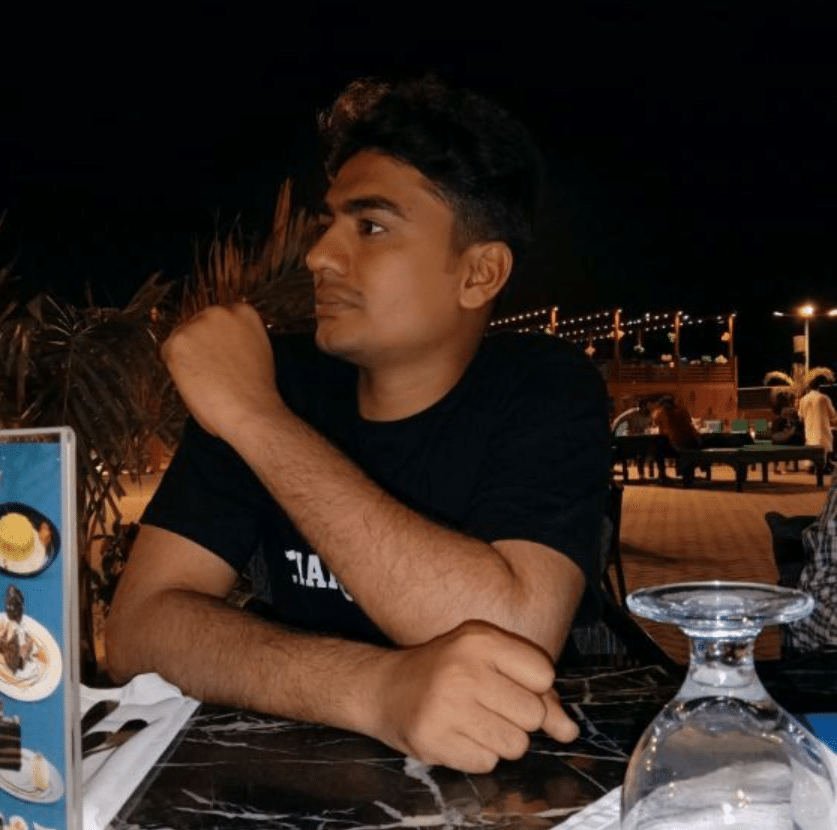
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.