The code snippet helps you to create a loading progress bar animation on HTML5 canvas. It visualizes progress dynamically. A progress bar fills as content loads, offering a visual loading indicator.
Apply this code in web projects to enhance user experience during content loading on a canvas. Benefit: Provides a visually engaging loading indication for a smoother user interaction.
How to Create HTML5 Canvas Loading Progress Bar Animation
1. First of all, create an HTML file. Add the necessary HTML structure. Include a <canvas></canvas>
tag.
<canvas></canvas>
2. Integrate the given CSS code within <style>
tags or link an external CSS file. Adjust the canvas position and layout as per your page requirements.
body{ margin: 0; padding: 0; background: #272822; } canvas{ position: absolute; top: calc(50% - 50px); top: -webkit-calc(50% - 100px); left: calc(50% - 200px); left: -webkit-calc(50% - 200px); }
3. Load the jQuery JavaScript library by adding the following CDN link just before closing the <body> element:
<script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js'></script>
4. Finally, include the following JavaScript code in a <script>
tag or link an external JS file. This code initializes and manages the loading progress animation.
Adjust variables like particle_no
to change the number of particles in the animation. Modify colors, dimensions, or animation behavior according to your design preferences.
particle_no = 25; window.requestAnimFrame = (function() { return window.requestAnimationFrame || window.webkitRequestAnimationFrame || window.mozRequestAnimationFrame || window.oRequestAnimationFrame || window.msRequestAnimationFrame || function(callback) { window.setTimeout(callback, 1000 / 60); }; })(); var canvas = document.getElementsByTagName("canvas")[0]; var ctx = canvas.getContext("2d"); var counter = 0; var particles = []; var w = 400, h = 200; canvas.width = w; canvas.height = h; function reset() { ctx.fillStyle = "#272822"; ctx.fillRect(0, 0, w, h); ctx.fillStyle = "#171814"; ctx.fillRect(25, 80, 350, 25); } function progressbar() { this.widths = 0; this.hue = 0; this.draw = function() { ctx.fillStyle = 'hsla(' + this.hue + ', 100%, 40%, 1)'; ctx.fillRect(25, 80, this.widths, 25); var grad = ctx.createLinearGradient(0, 0, 0, 130); grad.addColorStop(0, "transparent"); grad.addColorStop(1, "rgba(0,0,0,0.5)"); ctx.fillStyle = grad; ctx.fillRect(25, 80, this.widths, 25); } } function particle() { this.x = 23 + bar.widths; this.y = 82; this.vx = 0.8 + Math.random() * 1; this.v = Math.random() * 5; this.g = 1 + Math.random() * 3; this.down = false; this.draw = function() { ctx.fillStyle = 'hsla(' + (bar.hue + 0.3) + ', 100%, 40%, 1)';; var size = Math.random() * 2; ctx.fillRect(this.x, this.y, size, size); } } bar = new progressbar(); function draw() { reset(); counter++ bar.hue += 0.8; bar.widths += 2; if (bar.widths > 350) { if (counter > 215) { reset(); bar.hue = 0; bar.widths = 0; counter = 0; particles = []; } else { bar.hue = 126; bar.widths = 351; bar.draw(); } } else { bar.draw(); for (var i = 0; i < particle_no; i += 10) { particles.push(new particle()); } } update(); } function update() { for (var i = 0; i < particles.length; i++) { var p = particles[i]; p.x -= p.vx; if (p.down == true) { p.g += 0.1; p.y += p.g; } else { if (p.g < 0) { p.down = true; p.g += 0.1; p.y += p.g; } else { p.y -= p.g; p.g -= 0.1; } } p.draw(); } } function animloop() { draw(); requestAnimFrame(animloop); } animloop();
Feel free to customize it to suit your design and enhance the user experience during content loading.
That’s all! hopefully, you have successfully created a Canvas Loading Progress Bar Animation. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
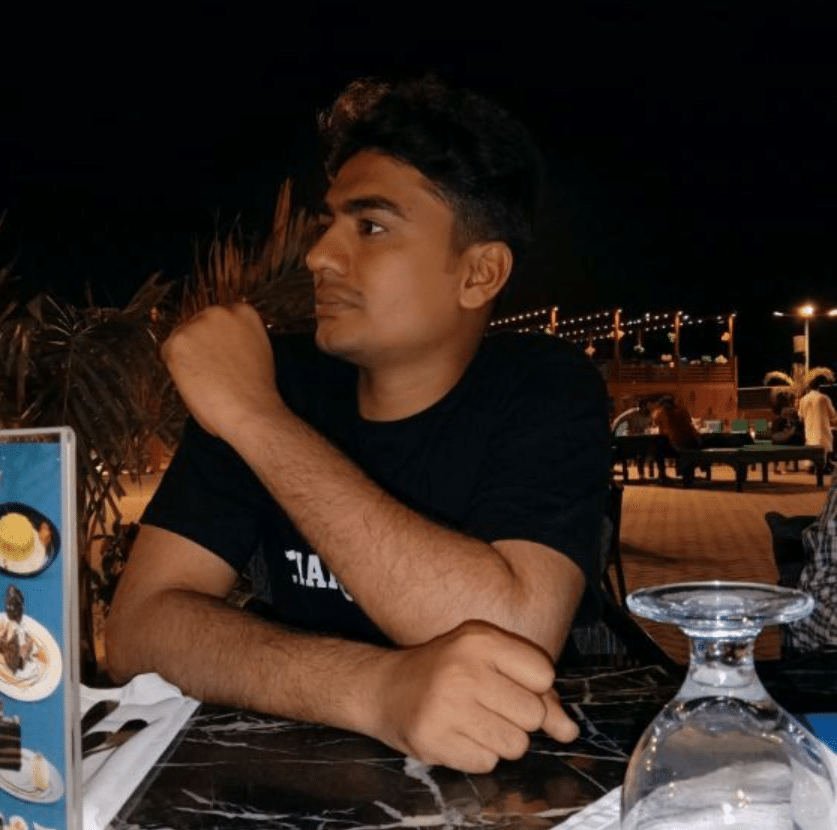
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.