This JavaScript code snippet helps you to create a swipe list menu to reveal list options by swiping. It uses touchmove function to detect swipes and display options behind a list.
How to Create Swipe List Menu in JavaScript
1. First of all, create the HTML structure as follows:
<ul> <li> <div class="item"> <svg viewBox="0 0 528.899 528.899"> <path fill="white" d="M328.883,89.125l107.59,107.589l-272.34,272.34L56.604,361.465L328.883,89.125z M518.113,63.177l-47.981-47.981 c-18.543-18.543-48.653-18.543-67.259,0l-45.961,45.961l107.59,107.59l53.611-53.611 C532.495,100.753,532.495,77.559,518.113,63.177z M0.3,512.69c-1.958,8.812,5.998,16.708,14.811,14.565l119.891-29.069 L27.473,390.597L0.3,512.69z" /> </svg> <svg viewBox="0 0 515.556 515.556"> <path d="m64.444 451.111c0 35.526 28.902 64.444 64.444 64.444h257.778c35.542 0 64.444-28.918 64.444-64.444v-322.222h-386.666z" /> <path d="m322.222 32.222v-32.222h-128.889v32.222h-161.111v64.444h451.111v-64.444z" /> </svg> <div class="overlay">Swipe to Right & Left</div> </div> </li> <li> <div class="item"> <svg viewBox="0 0 528.899 528.899"> <path fill="white" d="M328.883,89.125l107.59,107.589l-272.34,272.34L56.604,361.465L328.883,89.125z M518.113,63.177l-47.981-47.981 c-18.543-18.543-48.653-18.543-67.259,0l-45.961,45.961l107.59,107.59l53.611-53.611 C532.495,100.753,532.495,77.559,518.113,63.177z M0.3,512.69c-1.958,8.812,5.998,16.708,14.811,14.565l119.891-29.069 L27.473,390.597L0.3,512.69z" /> </svg> <svg viewBox="0 0 515.556 515.556"> <path d="m64.444 451.111c0 35.526 28.902 64.444 64.444 64.444h257.778c35.542 0 64.444-28.918 64.444-64.444v-322.222h-386.666z" /> <path d="m322.222 32.222v-32.222h-128.889v32.222h-161.111v64.444h451.111v-64.444z" /> </svg> <div class="overlay">Swipe to Right & Left</div> </div> </li> <li> <div class="item"> <svg viewBox="0 0 528.899 528.899"> <path fill="white" d="M328.883,89.125l107.59,107.589l-272.34,272.34L56.604,361.465L328.883,89.125z M518.113,63.177l-47.981-47.981 c-18.543-18.543-48.653-18.543-67.259,0l-45.961,45.961l107.59,107.59l53.611-53.611 C532.495,100.753,532.495,77.559,518.113,63.177z M0.3,512.69c-1.958,8.812,5.998,16.708,14.811,14.565l119.891-29.069 L27.473,390.597L0.3,512.69z" /> </svg> <svg viewBox="0 0 515.556 515.556"> <path d="m64.444 451.111c0 35.526 28.902 64.444 64.444 64.444h257.778c35.542 0 64.444-28.918 64.444-64.444v-322.222h-386.666z" /> <path d="m322.222 32.222v-32.222h-128.889v32.222h-161.111v64.444h451.111v-64.444z" /> </svg> <div class="overlay">Swipe to Right & Left</div> </div> </li> <li> <div class="item"> <svg viewBox="0 0 528.899 528.899"> <path fill="white" d="M328.883,89.125l107.59,107.589l-272.34,272.34L56.604,361.465L328.883,89.125z M518.113,63.177l-47.981-47.981 c-18.543-18.543-48.653-18.543-67.259,0l-45.961,45.961l107.59,107.59l53.611-53.611 C532.495,100.753,532.495,77.559,518.113,63.177z M0.3,512.69c-1.958,8.812,5.998,16.708,14.811,14.565l119.891-29.069 L27.473,390.597L0.3,512.69z" /> </svg> <svg viewBox="0 0 515.556 515.556"> <path d="m64.444 451.111c0 35.526 28.902 64.444 64.444 64.444h257.778c35.542 0 64.444-28.918 64.444-64.444v-322.222h-386.666z" /> <path d="m322.222 32.222v-32.222h-128.889v32.222h-161.111v64.444h451.111v-64.444z" /> </svg> <div class="overlay">Swipe to Right & Left</div> </div> </li> </ul>
2. After that, add the following CSS styles to your project:
body{ font-family: 'Verdana'; font-size: 14px; } main div{ width: 300px; height: 40px; box-sizing: border-box; padding: 10px 15px; color: white; } ul{ list-style: none; width: 300px; padding: 0px; margin: 0px auto; } ul li:not(:last-child){ margin-bottom: 10px; } ul li .item{ background: black; position: relative; overflow: hidden; } ul li .item svg{ width: 15px; height: 15px; } ul li .item svg:nth-child(2){ margin-left: 15px; } ul li .item svg path{ fill: white; } ul li .item .overlay{ position: absolute; top: 0px; left: 0px; background: #2f3d9a; transition: all 0.5s; }
3. Finally, add the following JavaScript code and done.
var items = document.querySelectorAll('.overlay'); items.forEach(item => { item.addEventListener('touchstart', handleTouchStart, false); item.addEventListener('touchmove', handleTouchMove, false); }); var xDown = null; var yDown = null; function getTouches(evt) { return evt.touches || evt.originalEvent.touches; } function handleTouchStart(evt) { const firstTouch = getTouches(evt)[0]; xDown = firstTouch.clientX; yDown = firstTouch.clientY; elm = firstTouch.target; }; function handleTouchMove(evt) { if ( ! xDown || ! yDown ) { return; } var xUp = evt.touches[0].clientX; var yUp = evt.touches[0].clientY; var xDiff = xDown - xUp; if ( xDiff > 0 ) { elm.style.left = '0px'; } else { elm.style.left = '80px'; } xDown = null; yDown = null; };
That’s all! hopefully, you have successfully integrated this swipe list menu code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
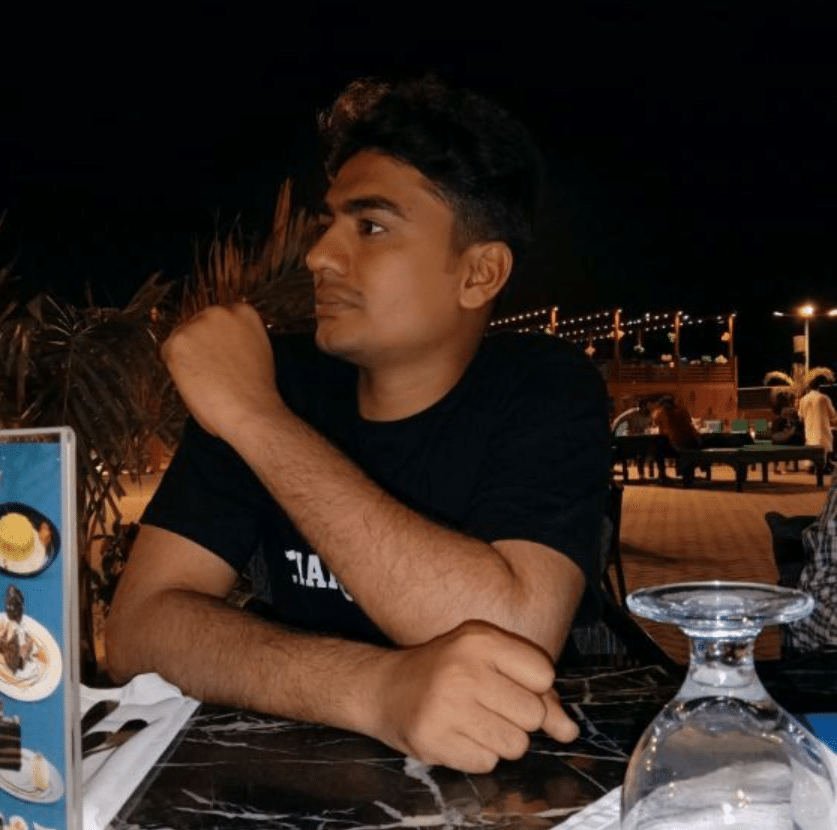
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.