This JavaScript and CSS code snippet helps you to create light/dark theme toggle functionality for your website. It comes with a checkbox toggle button that switch between light and dark mode. The JavaScript function detects the checkbox checked property and add an attribute “data-theme” to the root of document with two possible values, “light” and “dark”. The CSS contains the styles for the dark and light mode in variables. So, when a user checked the checkbox, the dark mode will be activated.
How to Create Light/Dark Theme Toggle with CSS and JavaScript
1. First of all, create an input element with a unique id “switch” and define its name attribute with “theme” value. Similarly, create a label tag associated with it, wrap both elements into a div tag and define its class name “toggle-container”. You can place this toggle button anywhere in your HTML where you want to display a light/dark switch button.
- <div class="container">
- <h1>Light / Dark Mode</h1>
- <div class="toggle-container">
- <input type="checkbox" id="switch" name="theme" /><label for="switch">Toggle</label>
- </div>
- <p>CodeHim is one of the best developer websites that provide web designers and developers with a simple way to view and download a variety of HTML, CSS, and JavaScript code snippets.</p>
- </div>
2. Now, add the following CSS styles to your project. You can modify the styles for the html selector according to your webpage.
- html {
- height: 100%;
- font-family: "Montserrat";
- display: grid;
- align-items: center;
- justify-items: center;
- --bg: #FCFCFC;
- --bg-panel: #EBEBEB;
- --color-headings: #0077FF;
- --color-text: #333333;
- }
- html[data-theme=dark] {
- --bg: #333333;
- --bg-panel: #434343;
- --color-headings: #3694FF;
- --color-text: #B5B5B5;
- }
- body {
- background-color: var(--bg);
- }
- .container {
- background-color: var(--bg-panel);
- margin: 5em;
- padding: 5em;
- border-radius: 15px;
- display: grid;
- grid-template-columns: 80% auto;
- grid-template-rows: auto auto;
- grid-template-areas: "title switch" "content content";
- }
- .container h1 {
- margin: 0;
- color: var(--color-headings);
- }
- .container p {
- color: var(--color-text);
- grid-area: content;
- font-size: 1.1em;
- line-height: 1.8em;
- margin-top: 2em;
- }
- input[type=checkbox] {
- height: 0;
- width: 0;
- visibility: hidden;
- }
- label {
- cursor: pointer;
- text-indent: -9999px;
- width: 52px;
- height: 27px;
- background: grey;
- float: right;
- border-radius: 100px;
- position: relative;
- }
- label:after {
- content: "";
- position: absolute;
- top: 3px;
- left: 3px;
- width: 20px;
- height: 20px;
- background: #fff;
- border-radius: 90px;
- transition: 0.3s;
- }
- input:checked + label {
- background: var(--color-headings);
- }
- input:checked + label:after {
- left: calc(100% - 5px);
- transform: translateX(-100%);
- }
- label:active:after {
- width: 45px;
- }
- html.transition,
- html.transition *,
- html.transition *:before,
- html.transition *:after {
- transition: all 750ms !important;
- transition-delay: 0 !important;
- }
3. Finally, add the following JavaScript function before closing of the body tag.
- var checkbox = document.querySelector('input[name=theme]');
- checkbox.addEventListener('change', function() {
- if(this.checked) {
- trans()
- document.documentElement.setAttribute('data-theme', 'dark')
- } else {
- trans()
- document.documentElement.setAttribute('data-theme', 'light')
- }
- })
- let trans = () => {
- document.documentElement.classList.add('transition');
- window.setTimeout(() => {
- document.documentElement.classList.remove('transition')
- }, 1000)
- }
That’s all! hopefully, you have successfully created light/dark theme toggle with CSS and JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
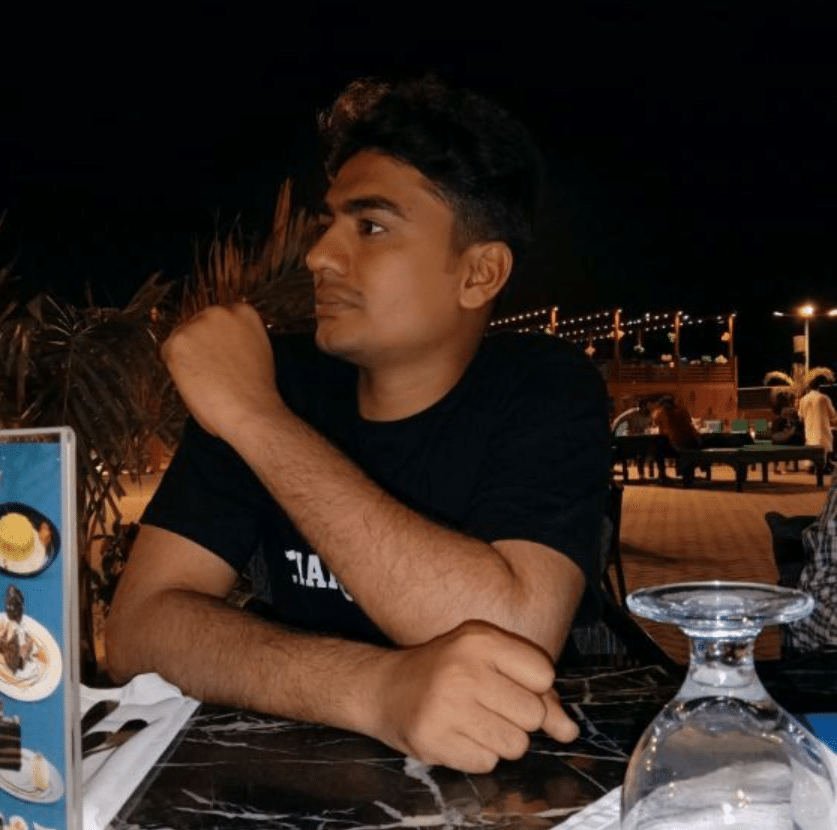
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.