This lightweight JavaScript code snippet helps you to create table search box for all columns. It filters whole table data on keyup function using indexof method. You can integrate into your project to make a quick search functionality for HTML table element.
How to Create JavaScript Table Search All Columns
1. First of all, create the HTML structure as follows:
<h2>My Customers</h2> <input type="text" id="myInput" onkeyup="myFunction()" placeholder="Search for names.." title="Type in a name"> <table id="myTable"> <tr class="header"> <th style="width:60%;">Name</th> <th style="width:40%;">Country</th> </tr> <tr> <td>Alfreds Futterkiste</td> <td>Germany</td> </tr> <tr> <td>Berglunds snabbkop</td> <td>Sweden</td> </tr> <tr> <td>Island Trading</td> <td>UK</td> </tr> <tr> <td>Koniglich Essen</td> <td>Germany</td> </tr> <tr> <td>Laughing Bacchus Winecellars</td> <td>Canada</td> </tr> <tr> <td>Magazzini Alimentari Riuniti</td> <td>Italy</td> </tr> <tr> <td>North/South</td> <td>UK</td> </tr> <tr> <td>Paris specialites</td> <td>France</td> </tr> </table>
2. After that, add the following CSS styles to your project:
* { box-sizing: border-box; } #myInput { background-image: url('/css/searchicon.png'); background-position: 10px 10px; background-repeat: no-repeat; width: 100%; font-size: 16px; padding: 12px 20px 12px 40px; border: 1px solid #ddd; margin-bottom: 12px; } #myTable { border-collapse: collapse; width: 100%; border: 1px solid #ddd; font-size: 18px; } #myTable th, #myTable td { text-align: left; padding: 12px; } #myTable tr { border-bottom: 1px solid #ddd; } #myTable tr.header, #myTable tr:hover { background-color: #f1f1f1; }
3. Finally, add the following JavaScript code and done.
function myFunction() { var input, filter, table, tr, td, i; input = document.getElementById("myInput"); filter = input.value.toUpperCase(); table = document.getElementById("myTable"); tr = table.getElementsByTagName("tr"); for (i = 0; i < tr.length; i++) { td = tr[i].getElementsByTagName("td")[0]; if (td) { if (td.innerHTML.toUpperCase().indexOf(filter) > -1) { tr[i].style.display = ""; } else { tr[i].style.display = "none"; } } } }
That’s all! hopefully, you have successfully integrated this table search code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
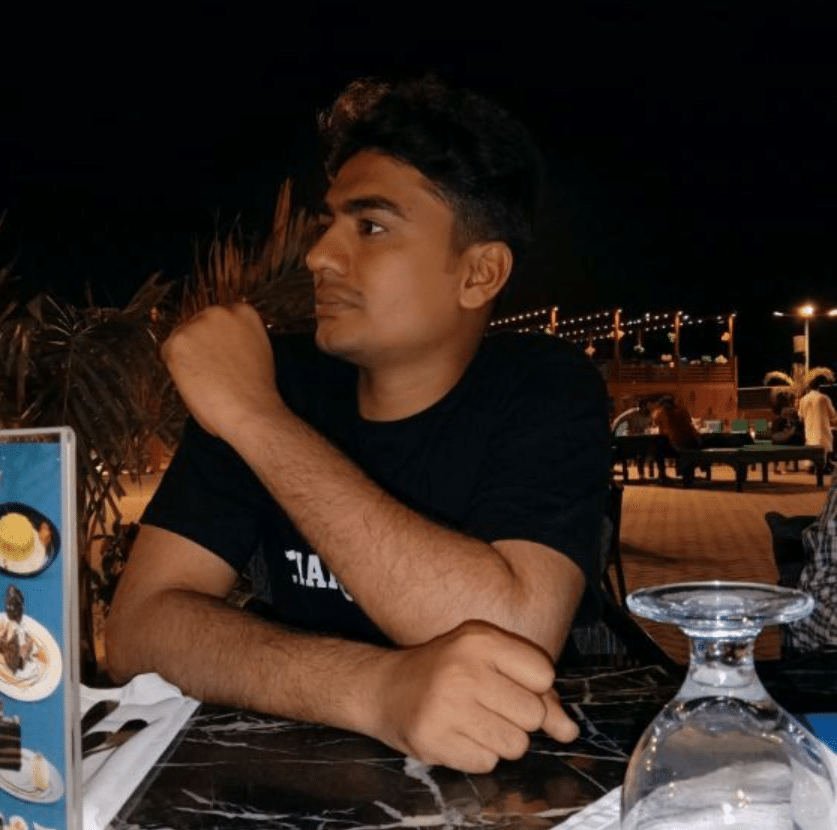
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.