This code creates a 3D image slideshow using HTML and CSS. It arranges images in a rotating manner to create a slideshow effect. The JavaScript helps in cycling through the images continuously. It helps display images dynamically in a visually appealing manner.
You can use this code on websites or projects needing a visually engaging image slideshow. It’s great for showcasing products or creating captivating image galleries that grab attention. The benefit? It offers an interactive way to display multiple images seamlessly.
How to Create a 3D Image Slideshow Using HTML and CSS
1. Create a container <div>
in your HTML file and add multiple <div>
elements with the class “box” inside it. Each “box” will hold an image. Replace the placeholder image URLs in the HTML with the URLs of your own images.
<div class="img-container"> <div class="box"> <img src="https://img.freepik.com/free-photo/wide-angle-shot-single-tree-growing-clouded-sky-during-sunset-surrounded-by-grass_181624-22807.jpg?w=740&t=st=1692617800~exp=1692618400~hmac=da967fd7227c9ad38cd8a070b995acb5f4bb6c2185c6a20bd2e90c60e2abf6ab" alt="" /> </div> <div class="box"> <img src="https://img.freepik.com/free-photo/wide-angle-shot-single-tree-growing-clouded-sky-during-sunset-surrounded-by-grass_181624-22807.jpg?w=740&t=st=1692617800~exp=1692618400~hmac=da967fd7227c9ad38cd8a070b995acb5f4bb6c2185c6a20bd2e90c60e2abf6ab" alt="" /> </div> <div class="box"> <img src="https://img.freepik.com/free-photo/beautiful-view-greenery-bridge-forest-perfect-background_181624-17827.jpg?w=740&t=st=1692619142~exp=1692619742~hmac=cbfd96c2635889371b9d74ff41432f9944fadfef177e121f43cf9f2f94b2fd6a" alt="" /> </div> <div class="box"> <img src="https://img.freepik.com/free-photo/seljalandsfoss-waterfall-during-sunset-beautiful-waterfall-iceland_335224-596.jpg?w=740&t=st=1692619874~exp=1692620474~hmac=c48ffd5f0d2418bb4600d98ac64f3f61a0ed9e2090751ac7fa32bff35687ee17" alt="" /> </div> <div class="box"> <img src="https://img.freepik.com/free-photo/landscape-morning-fog-mountains-with-hot-air-balloons-sunrise_335224-794.jpg?w=740&t=st=1692619958~exp=1692620558~hmac=d2e0e7c3c55857d0bb617b4b5b4deb0c7c67c6677c1eaa8b4c73d50445ece5bf" alt="" /> </div> <div class="box"> <img src="https://img.freepik.com/free-photo/wide-angle-shot-single-tree-growing-clouded-sky-during-sunset-surrounded-by-grass_181624-22807.jpg?w=740&t=st=1692617800~exp=1692618400~hmac=da967fd7227c9ad38cd8a070b995acb5f4bb6c2185c6a20bd2e90c60e2abf6ab" alt="" /> </div> </div>
2. Use CSS to style the image container and boxes to achieve the 3D effect and positioning. Adjust the CSS properties like size, positioning, transitions, and rotations to fit your design preferences.
* { margin: 0; padding: 0; box-sizing: border-box; user-select: none; } body { height: 100vh; background-color: #f5f5f5; display: flex; align-items: center; justify-content: center; } .img-container { position: relative; height: 500px; width: 700px; perspective: 500px; transform-style: preserve-3d; } .box { box-shadow: 0 0 10px rgb(0 0 0 / 30%); width: 600px; height: 440px; border-radius: 25px; overflow: hidden; border: 1px solid #bbb; position: absolute; top: 50%; transition: 800ms ease-in-out; } .box img { width: 100%; height: 100%; } .box:first-of-type { z-index: 5; opacity: 0; left: 15%; transform: translate(-50%, -50%) rotateY(-10deg); } .box:nth-of-type(2) { opacity: 1; left: 20%; transform: translate(-50%, -50%) rotateY(-10deg); z-index: 5; } .box:nth-of-type(3) { left: 25%; opacity: 0.75; color: #eee; z-index: 10; transform: translate(-50%, -50%) rotateY(-10deg) translateZ(-50px); } .box:nth-of-type(4) { z-index: 5; opacity: 0.5; left:30%; transform: translate(-50%, -50%) rotateY(-10deg) translateZ(-100px); } .box:nth-of-type(5) { z-index: 2; opacity: 0.25; left: 35%; transform: translate(-50%, -50%) rotateY(-10deg) translateZ(-150px); } .box:nth-of-type(6) { z-index: 2; opacity: 0.0; left: 40%; transform: translate(-50%, -50%) rotateY(-10deg) translateZ(-200px); }
3. Finally, include the JavaScript code to enable the slideshow functionality.
let imgContainer = document.querySelector(".img-container"); setInterval(() => { let last = imgContainer.firstElementChild; last.remove(); imgContainer.appendChild(last); }, 2500);
That’s all! hopefully, you have successfully created a 3D Image Slideshow on your website. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
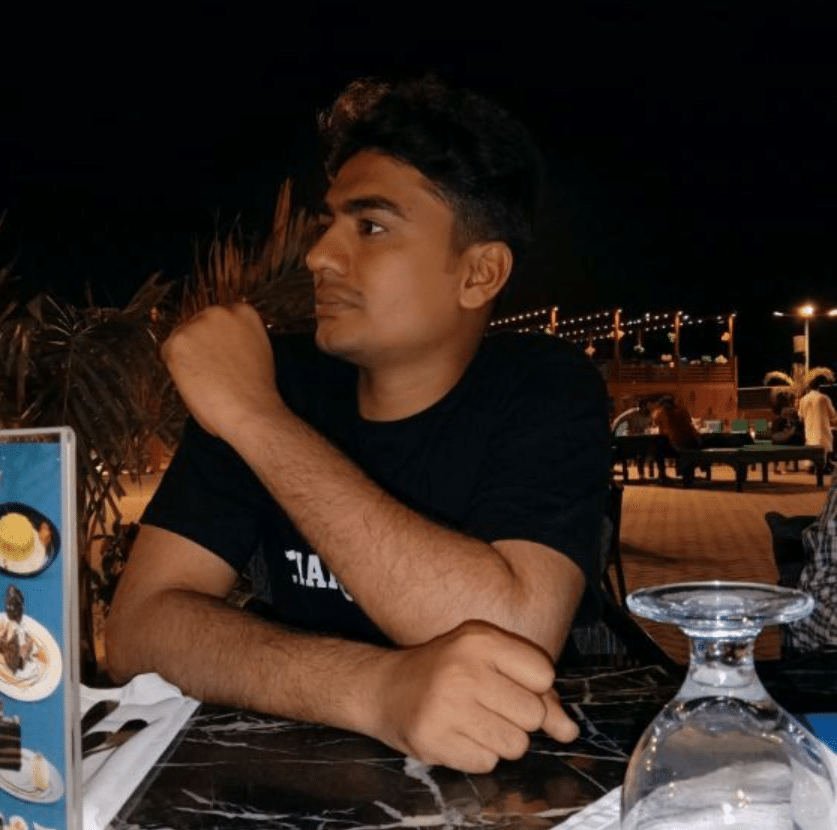
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.