This JavaScript code snippet offers functionality to change the color of the website’s navbar on the scroll event. This effect is achieved by adding a "scrolled"
class to the navigation bar element when the page is scrolled.
After a one-second delay, the code checks if the user has scrolled back to the top, then makes the navigation bar transparent again, creating a sleek scrolling experience for the website visitors.
How to Change Navbar Color On Scroll Using JavaScript
1. First, create your HTML structure. You’ll need a basic HTML structure with a header that contains your navigation bar and a main content section. Here’s an example:
<header> <nav id="navigation"> <ul> <li>Link One</li> <li>Link Two</li> <li>Link Three</li> </ul> </nav> </header> <main> <!-- Your contetn goes here --> </main>
2. Next, let’s style the elements. In this example, we’re using a CSS file (styles.css) to style the header, navigation bar, and main content section. We’ll also define the "scrolled"
class that will change the navigation bar’s background color when added.
*, *::before, *::after { padding: 0; margin: 0; box-sizing: border-box; } html { width: 100%; height: 100vh; } body { font-family: -apple-system, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; font-size: 18px; } header { position: sticky; top: 0; } nav { background: transparent; transition: all ease 500ms; } nav ul { display: flex; align-items: center; justify-content: center; list-style: none; } nav ul li { padding: 2rem; margin: 1rem; text-transform: uppercase; font-weight: bold; } h1 { font-size: 3.6rem; text-align: center; } main { width: 80vw; margin: 2rem auto; } main div { margin: 2rem; } .scrolled { background: hsl(160, 100%, 50%); box-shadow: 0 1px 5px hsla(0, 0%, 0%, 0.3); }
4. Now, let’s add the JavaScript code (script.js) that will change the navigation bar’s color as you scroll down the page.
// Get the Navbar element const nav = document.getElementById('navigation'); // Add an event listener to the document // so that a class is added to the nav // element when the page is scrolled document.addEventListener('scroll', () => { nav.classList.add('scrolled'); // After 1s, check if the user has scrolled to // the top of the page and make the nav // element transparent again setTimeout(() => { if (window.pageYOffset === 0 && nav.classList.contains('scrolled')) { nav.classList.remove('scrolled'); } }, 1000); });
That’s all! hopefully, You’ve successfully created a simple and visually appealing scroll effect for your web page’s navigation bar using HTML, CSS, and JavaScript. Feel free to customize the styles and timing to fit your website’s design.
If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
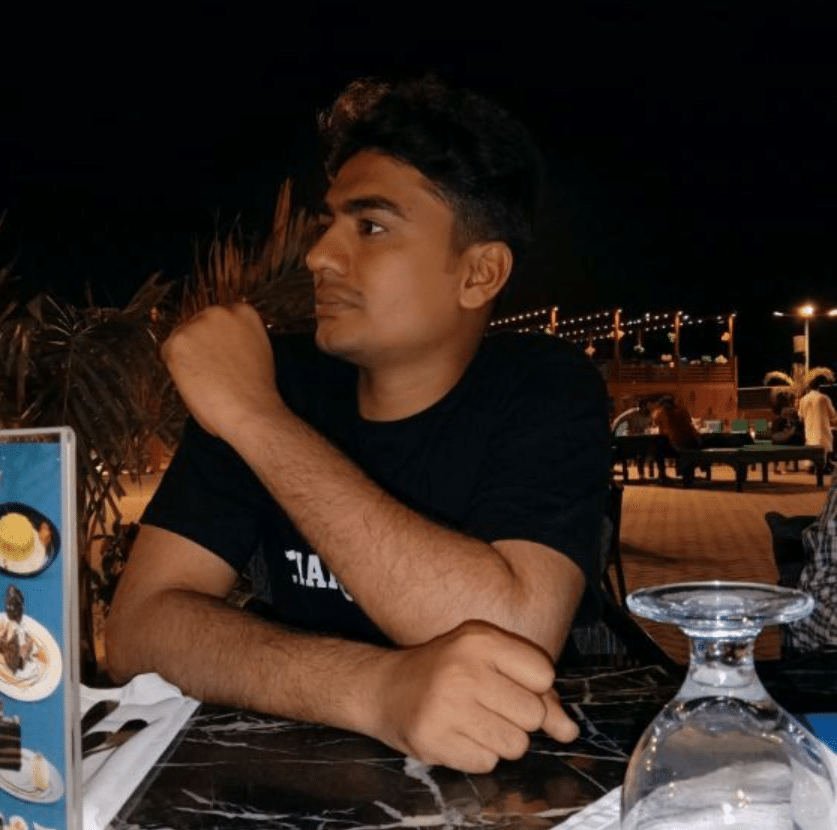
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.