This code allows you to expand and collapse table rows in HTML and CSS. It works by toggling row visibility when clicked. This functionality is helpful for managing content visibility in tables.
You can use this code on websites with tables to make them interactive, allowing users to expand and collapse rows. It enhances user experience by providing a more organized view of data. It’s easy to implement and improves table readability.
How to Create Expand and Collapse Table Rows in HTML CSS
1. First, in the <head>
section of your HTML file, link the Modernizr JS, Normalize CSS, Prefixfree JS, and jQuery. You can host these files locally or use external resources like CDNs.
<script src="https://cdnjs.cloudflare.com/ajax/libs/modernizr/2.8.3/modernizr.min.js" type="text/javascript"></script> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css"> <script src="https://cdnjs.cloudflare.com/ajax/libs/prefixfree/1.0.7/prefixfree.min.js"></script> <script src='//cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js'></script>
2. Create the HTML table structure that you want to make expandable and collapsible. Make sure to include the necessary HTML elements such as <table>
, <thead>
, <tbody>
, <tr>
, <th>
, and <td>
. Add CSS classes to your table rows to identify which rows should be expandable and collapsible. In the provided code, the class “view” is used for this purpose.
<table class="fold-table"> <thead> <tr> <th>Company</th><th>Amount</th><th>Value</th><th>Premiums</th><th>Strategy A</th><th>Strategy B</th><th>Strategy C</th> </tr> </thead> <tbody> <tr class="view"> <td>Company Name</td> <td class="pcs">457</td> <td class="cur">6535178</td> <td>-</td> <td class="per">50,71</td> <td class="per">49,21</td> <td class="per">0</td> </tr> <tr class="fold"> <td colspan="7"> <div class="fold-content"> <h3>Company Name</h3> <p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas.</p> <table> <thead> <tr> <th>Company name</th><th>Customer no</th><th>Customer name</th><th>Insurance no</th><th>Strategy</th><th>Start</th><th>Current</th><th>Diff</th> </tr> </thead> <tbody> <tr> <td>Sony</td> <td>13245</td> <td>John Doe</td> <td>064578</td> <td>A, 100%</td> <td class="cur">20000</td> <td class="cur">33000</td> <td class="cur">13000</td> </tr> <tr> <td>Sony</td> <td>13288</td> <td>Claire Bennet</td> <td>064877</td> <td>B, 100%</td> <td class="cur">28000</td> <td class="cur">48000</td> <td class="cur">20000</td> </tr> <tr> <td>Sony</td> <td>12341</td> <td>Barry White</td> <td>064123</td> <td>A, 100%</td> <td class="cur">10000</td> <td class="cur">22000</td> <td class="cur">12000</td> </tr> </tbody> </table> </div> </td> </tr> <tr class="view"> <td>Company Name</td> <td class="pcs">457</td> <td class="cur">6535178</td> <td>-</td> <td class="per">50,71</td> <td class="per">49,21</td> <td class="per">0</td> </tr> <tr class="fold"> <td colspan="7"> <div class="fold-content"> <h3>Company Name</h3> <p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas.</p> <table> <thead> <tr> <th>Company name</th><th>Customer no</th><th>Customer name</th><th>Insurance no</th><th>Strategy</th><th>Start</th><th>Current</th><th>Diff</th> </tr> </thead> <tbody> <tr> <td>Sony</td> <td>13245</td> <td>John Doe</td> <td>064578</td> <td>A, 100%</td> <td class="cur">20000</td> <td class="cur">33000</td> <td class="cur">13000</td> </tr> <tr> <td>Sony</td> <td>13288</td> <td>Claire Bennet</td> <td>064877</td> <td>B, 100%</td> <td class="cur">28000</td> <td class="cur">48000</td> <td class="cur">20000</td> </tr> <tr> <td>Sony</td> <td>12341</td> <td>Barry White</td> <td>064123</td> <td>A, 100%</td> <td class="cur">10000</td> <td class="cur">22000</td> <td class="cur">12000</td> </tr> </tbody> </table> </div> </td> </tr> <tr class="view"> <td>Company Name</td> <td class="pcs">457</td> <td class="cur">6535178</td> <td>-</td> <td class="per">50,71</td> <td class="per">49,21</td> <td class="per">0</td> </tr> <tr class="fold"> <td colspan="7"> <div class="fold-content"> <h3>Company Name</h3> <p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas.</p> <table> <thead> <tr> <th>Company name</th><th>Customer no</th><th>Customer name</th><th>Insurance no</th><th>Strategy</th><th>Start</th><th>Current</th><th>Diff</th> </tr> </thead> <tbody> <tr> <td>Sony</td> <td>13245</td> <td>John Doe</td> <td>064578</td> <td>A, 100%</td> <td class="cur">20000</td> <td class="cur">33000</td> <td class="cur">13000</td> </tr> <tr> <td>Sony</td> <td>13288</td> <td>Claire Bennet</td> <td>064877</td> <td>B, 100%</td> <td class="cur">28000</td> <td class="cur">48000</td> <td class="cur">20000</td> </tr> <tr> <td>Sony</td> <td>12341</td> <td>Barry White</td> <td>064123</td> <td>A, 100%</td> <td class="cur">10000</td> <td class="cur">22000</td> <td class="cur">12000</td> </tr> </tbody> </table> </div> </td> </tr> <tr class="view"> <td>Company Name</td> <td class="pcs">457</td> <td class="cur">6535178</td> <td>-</td> <td class="per">50,71</td> <td class="per">49,21</td> <td class="per">0</td> </tr> <tr class="fold"> <td colspan="7"> <div class="fold-content"> <h3>Company Name</h3> <p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas.</p> <table> <thead> <tr> <th>Company name</th><th>Customer no</th><th>Customer name</th><th>Insurance no</th><th>Strategy</th><th>Start</th><th>Current</th><th>Diff</th> </tr> </thead> <tbody> <tr> <td>Sony</td> <td>13245</td> <td>John Doe</td> <td>064578</td> <td>A, 100%</td> <td class="cur">20000</td> <td class="cur">33000</td> <td class="cur">13000</td> </tr> <tr> <td>Sony</td> <td>13288</td> <td>Claire Bennet</td> <td>064877</td> <td>B, 100%</td> <td class="cur">28000</td> <td class="cur">48000</td> <td class="cur">20000</td> </tr> <tr> <td>Sony</td> <td>12341</td> <td>Barry White</td> <td>064123</td> <td>A, 100%</td> <td class="cur">10000</td> <td class="cur">22000</td> <td class="cur">12000</td> </tr> </tbody> </table> </div> </td> </tr> <tr class="view"> <td>Company Name</td> <td class="pcs">457</td> <td class="cur">6535178</td> <td>-</td> <td class="per">50,71</td> <td class="per">49,21</td> <td class="per">0</td> </tr> <tr class="fold"> <td colspan="7"> <div class="fold-content"> <h3>Company Name</h3> <p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas.</p> <table> <thead> <tr> <th>Company name</th><th>Customer no</th><th>Customer name</th><th>Insurance no</th><th>Strategy</th><th>Start</th><th>Current</th><th>Diff</th> </tr> </thead> <tbody> <tr> <td>Sony</td> <td>13245</td> <td>John Doe</td> <td>064578</td> <td>A, 100%</td> <td class="cur">20000</td> <td class="cur">33000</td> <td class="cur">13000</td> </tr> <tr> <td>Sony</td> <td>13288</td> <td>Claire Bennet</td> <td>064877</td> <td>B, 100%</td> <td class="cur">28000</td> <td class="cur">48000</td> <td class="cur">20000</td> </tr> <tr> <td>Sony</td> <td>12341</td> <td>Barry White</td> <td>064123</td> <td>A, 100%</td> <td class="cur">10000</td> <td class="cur">22000</td> <td class="cur">12000</td> </tr> </tbody> </table> </div> </td> </tr> <tr class="view"> <td>Company Name</td> <td class="pcs">457</td> <td class="cur">6535178</td> <td>-</td> <td class="per">50,71</td> <td class="per">49,21</td> <td class="per">0</td> </tr> <tr class="fold"> <td colspan="7"> <div class="fold-content"> <h3>Company Name</h3> <p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas.</p> <table> <thead> <tr> <th>Company name</th><th>Customer no</th><th>Customer name</th><th>Insurance no</th><th>Strategy</th><th>Start</th><th>Current</th><th>Diff</th> </tr> </thead> <tbody> <tr> <td>Sony</td> <td>13245</td> <td>John Doe</td> <td>064578</td> <td>A, 100%</td> <td class="cur">20000</td> <td class="cur">33000</td> <td class="cur">13000</td> </tr> <tr> <td>Sony</td> <td>13288</td> <td>Claire Bennet</td> <td>064877</td> <td>B, 100%</td> <td class="cur">28000</td> <td class="cur">48000</td> <td class="cur">20000</td> </tr> <tr> <td>Sony</td> <td>12341</td> <td>Barry White</td> <td>064123</td> <td>A, 100%</td> <td class="cur">10000</td> <td class="cur">22000</td> <td class="cur">12000</td> </tr> </tbody> </table> </div> </td> </tr> <tr class="view"> <td>Company Name</td> <td class="pcs">457</td> <td class="cur">6535178</td> <td>-</td> <td class="per">50,71</td> <td class="per">49,21</td> <td class="per">0</td> </tr> <tr class="fold"> <td colspan="7"> <div class="fold-content"> <h3>Company Name</h3> <p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas.</p> <table> <thead> <tr> <th>Company name</th><th>Customer no</th><th>Customer name</th><th>Insurance no</th><th>Strategy</th><th>Start</th><th>Current</th><th>Diff</th> </tr> </thead> <tbody> <tr> <td>Sony</td> <td>13245</td> <td>John Doe</td> <td>064578</td> <td>A, 100%</td> <td class="cur">20000</td> <td class="cur">33000</td> <td class="cur">13000</td> </tr> <tr> <td>Sony</td> <td>13288</td> <td>Claire Bennet</td> <td>064877</td> <td>B, 100%</td> <td class="cur">28000</td> <td class="cur">48000</td> <td class="cur">20000</td> </tr> <tr> <td>Sony</td> <td>12341</td> <td>Barry White</td> <td>064123</td> <td>A, 100%</td> <td class="cur">10000</td> <td class="cur">22000</td> <td class="cur">12000</td> </tr> </tbody> </table> </div> </td> </tr> <tr class="view"> <td>Company Name</td> <td class="pcs">457</td> <td class="cur">6535178</td> <td>-</td> <td class="per">50,71</td> <td class="per">49,21</td> <td class="per">0</td> </tr> <tr class="fold"> <td colspan="7"> <div class="fold-content"> <h3>Company Name</h3> <p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas.</p> <table> <thead> <tr> <th>Company name</th><th>Customer no</th><th>Customer name</th><th>Insurance no</th><th>Strategy</th><th>Start</th><th>Current</th><th>Diff</th> </tr> </thead> <tbody> <tr> <td>Sony</td> <td>13245</td> <td>John Doe</td> <td>064578</td> <td>A, 100%</td> <td class="cur">20000</td> <td class="cur">33000</td> <td class="cur">13000</td> </tr> <tr> <td>Sony</td> <td>13288</td> <td>Claire Bennet</td> <td>064877</td> <td>B, 100%</td> <td class="cur">28000</td> <td class="cur">48000</td> <td class="cur">20000</td> </tr> <tr> <td>Sony</td> <td>12341</td> <td>Barry White</td> <td>064123</td> <td>A, 100%</td> <td class="cur">10000</td> <td class="cur">22000</td> <td class="cur">12000</td> </tr> </tbody> </table> </div> </td> </tr> </tbody> </table>
Replace the sample data with your own data in the above HTML code.
3. Now, add the following CSS code to style the table. You can modify the CSS rules according to your website/app’s theme.
@charset "UTF-8"; @import url("https://netdna.bootstrapcdn.com/font-awesome/4.0.3/css/font-awesome.css"); .pcs:after { content: " pcs"; } .cur:before { content: "$"; } .per:after { content: "%"; } * { box-sizing: border-box; } body { padding: 0.2em 2em; } table { width: 100%; } table th { text-align: left; border-bottom: 1px solid #ccc; } table th, table td { padding: 0.4em; } table.fold-table > tbody > tr.view td, table.fold-table > tbody > tr.view th { cursor: pointer; } table.fold-table > tbody > tr.view td:first-child, table.fold-table > tbody > tr.view th:first-child { position: relative; padding-left: 20px; } table.fold-table > tbody > tr.view td:first-child:before, table.fold-table > tbody > tr.view th:first-child:before { position: absolute; top: 50%; left: 5px; width: 9px; height: 16px; margin-top: -8px; font: 16px fontawesome; color: #999; content: ""; transition: all 0.3s ease; } table.fold-table > tbody > tr.view:nth-child(4n-1) { background: #eee; } table.fold-table > tbody > tr.view:hover { background: #000; } table.fold-table > tbody > tr.view.open { background: tomato; color: white; } table.fold-table > tbody > tr.view.open td:first-child:before, table.fold-table > tbody > tr.view.open th:first-child:before { transform: rotate(-180deg); color: #333; } table.fold-table > tbody > tr.fold { display: none; } table.fold-table > tbody > tr.fold.open { display: table-row; } .fold-content { padding: 0.5em; } .fold-content h3 { margin-top: 0; } .fold-content > table { border: 2px solid #ccc; } .fold-content > table > tbody tr:nth-child(even) { background: #eee; }
4. Finally, add the JavaScript code to your project to toggle the visibility of table rows when a row with the “view” class is clicked. Ensure that you have jQuery included in your project, as the code uses it for event handling.
$(function(){ $(".fold-table tr.view").on("click", function(){ $(this).toggleClass("open").next(".fold").toggleClass("open"); }); });
That’s all! hopefully, you have successfully created a table with expand and collapse rows using HTML, CSS, and JS. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
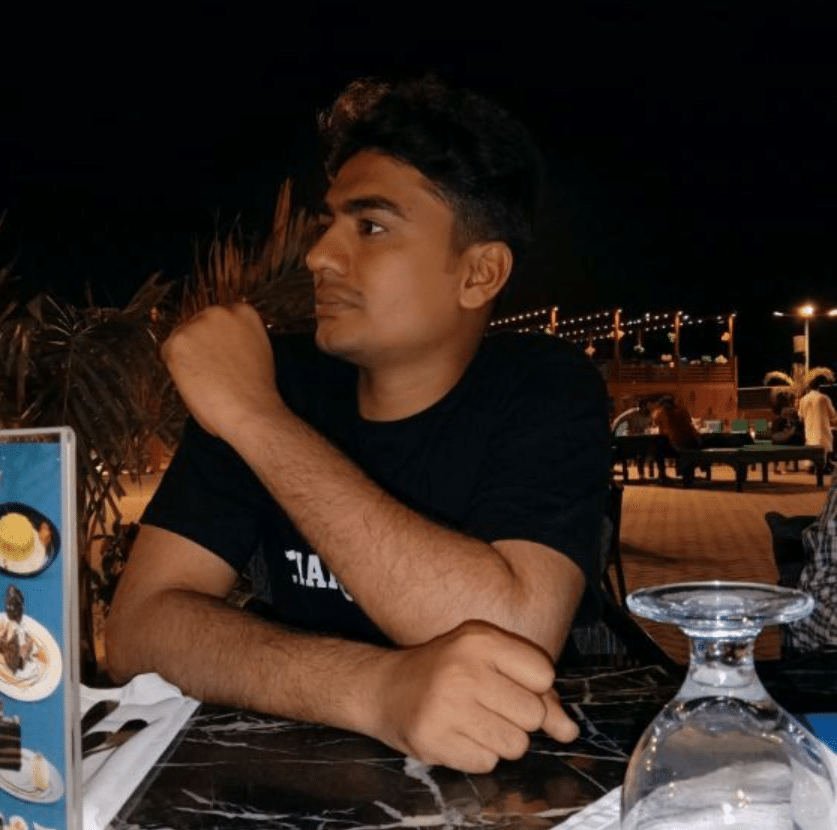
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.