This JavaScript code snippet helps you to create a single select dropdown. It handles select items through HTML5 data-attribute. So, you can add as many options as you want in a single select dropdown.
How to Create JavaScript Single Select Dropdown
1. First of all, create the HTML structure as follows:
- <div class="selects-box">
- <div class="select" data-select>
- <div class="select__selected-item" data-select-action data-select-open>
- <div class="select__selected-title" data-select-title>
- Choose item
- </div>
- <svg class="select__arrow" data-select-arrow width="24" height="24" viewBox="0 0 24 24" fill="none" xmlns="http://www.w3.org/2000/svg">
- <path d="M17 12L9 20L7 18L15 10" fill="#1E4DBA"/>
- <path d="M17 12L15 14L7 6L9 4" fill="#1E4DBA"/>
- </svg>
- </div>
- <div class="select__list" data-select-list>
- <div class="select__list-item" data-select-action data-select-item>CodeHim</div>
- <div class="select__list-item" data-select-action data-select-item>Free HTML code</div>
- <div class="select__list-item" data-select-action data-select-item>Web Designing</div>
- <div class="select__list-item" data-select-action data-select-item>Plugins</div>
- <div class="select__list-item" data-select-action data-select-item>Code Snippets</div>
- <div class="select__list-item" data-select-action data-select-item>Sixth item</div>
- <div class="select__list-item" data-select-action data-select-item>Eight item</div>
- <div class="select__list-item" data-select-action data-select-item>Ninth item</div>
- <div class="select__list-item" data-select-action data-select-item>Tenth item</div>
- </div>
- <input class="select__input" type="text" data-select-input>
- </div>
- </div>
2. After that, add the following CSS styles into your project:
- .selects-box {
- display: flex;
- flex-wrap: wrap;
- }
- .select {
- max-width: 280px;
- position: relative;
- width: 100%;
- margin: 20px;
- }
- .select__selected-item {
- background: #f4f4f4;
- padding: 10px 20px;
- border-radius: 4px;
- border: 2px solid #d8d8d8;
- color: #1e4dba;
- box-sizing: border-box;
- display: flex;
- align-items: center;
- justify-content: space-between;
- }
- .select__selected-item:hover {
- cursor: pointer;
- background: #f9f9f9;
- }
- .select__arrow {
- transition: 0.3s;
- }
- .select__arrow_rotate {
- transform: rotate(90deg);
- }
- .select__list {
- border: 2px solid transparent;
- border-radius: 4px;
- position: absolute;
- width: 100%;
- top: 50px;
- z-index: 1;
- box-sizing: border-box;
- transition: 0.3s;
- overflow: auto;
- max-height: 0;
- opacity: 0;
- }
- .select__list_opened {
- max-height: 200px;
- border-color: #d8d8d8;
- opacity: 1;
- }
- .select__list-item {
- padding: 10px 20px;
- color: #6f6f6f;
- border-bottom: 1px solid #d8d8d8;
- background: #fff;
- }
- .select__list-item:hover {
- text-decoration: underline;
- cursor: pointer;
- background: #f9f9f9;
- }
- .select__list-item:first-child {
- border-radius: 2px 2px 0 0;
- }
- .select__list-item:last-child {
- border-bottom: none;
- border-radius: 0 0 2px 2px;
- }
- .select__input {
- display: none;
- }
3. Finally, add the following JavaScript code and done.
- const SELECT = '[data-select]'
- const SELECT_LIST = '[data-select-list]'
- const SELECT_ARROW = '[data-select-arrow]'
- const SELECT_ACTION = '[data-select-action]'
- const SELECT_TITLE = '[data-select-title]'
- const SELECT_INPUT = '[data-select-input]'
- const SELECT_ITEM = 'selectItem'
- const OPEN_SELECT = 'selectOpen'
- class Select {
- static attach() {
- const select = new Select()
- select.init()
- }
- init() {
- if (this.findSelect()) {
- this.applyListener()
- }
- }
- applyListener() {
- document.querySelector('*').addEventListener('click', e => {
- const element = e.target.closest(SELECT_ACTION)
- if (this.isCallSelectElement(element)) {
- if (this.isOpened()) {
- this.closeSelectList()
- } else {
- this.openSelectList()
- }
- }
- if (this.isCallSelectItemElement(element)) {
- this.addSelectedValue(element)
- }
- if (this.isCallSelectElement(element) !== true && this.selectOverlayIsClickedElement(element) !== true) {
- this.closeSelectList()
- }
- })
- }
- isCallSelectElement(element) {
- return element && OPEN_SELECT in element.dataset
- }
- isCallSelectItemElement(element) {
- return element && SELECT_ITEM in element.dataset
- }
- findSelect() {
- const select = document.querySelector(SELECT)
- if (select) {
- this.select = select
- this.selectList = this.select.querySelector(SELECT_LIST)
- this.selectArrow = this.select.querySelector(SELECT_ARROW)
- this.selectTitle = this.select.querySelector(SELECT_TITLE)
- this.selectInput = this.select.querySelector(SELECT_INPUT)
- return true
- }
- return false
- }
- isOpened() {
- return this.selectList.classList.contains('select__list_opened')
- }
- openSelectList() {
- this.selectList.classList.add('select__list_opened')
- this.selectArrow.classList.add('select__arrow_rotate')
- }
- closeSelectList() {
- this.selectList.classList.remove('select__list_opened')
- this.selectArrow.classList.remove('select__arrow_rotate')
- }
- addSelectedValue(element) {
- this.selectTitle.innerHTML = element.innerHTML
- this.selectInput.value = element.innerHTML
- }
- selectOverlayIsClickedElement(element) {
- return element && 'select' in element.dataset
- }
- }
- Select.attach()
That’s all! hopefully, you have successfully integrated this select dropdown code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
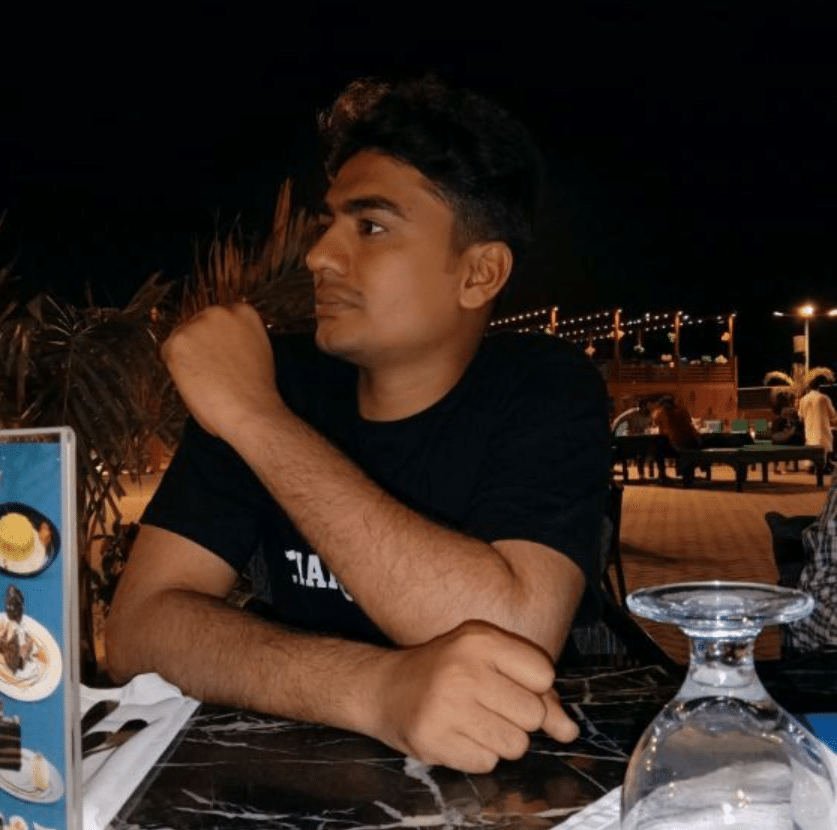
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.