This JavaScript Piano Keyboard App allows you to play musical notes using your computer keyboard. When you press a key on your keyboard, it triggers a corresponding musical note, and you can see the note displayed on the screen. The major functionality of this app is to provide a simple way for you to play musical notes and experiment with creating melodies using your computer keyboard.
It’s perfect for educational websites to teach music basics or for entertainment purposes, allowing users to play tunes with their keyboard. You can use this Piano App code on your website to create an interactive and fun musical experience for your users.
How to Create a JavaScript Piano Keyboard App
1. First, insert the HTML code into your web page where you want the piano keyboard to appear. Customize the app’s title and header as needed.
<section id="wrap"> <header> <h1>JS Piano</h1> <h2>Use your keyboard. Hover for hints.</h2> </header> <section id="main"> <div class="nowplaying"></div> <div class="keys"> <div data-key="65" class="key" data-note="C"> <span class="hints">A</span> </div> <div data-key="87" class="key sharp" data-note="C#"> <span class="hints">W</span> </div> <div data-key="83" class="key" data-note="D"> <span class="hints">S</span> </div> <div data-key="69" class="key sharp" data-note="D#"> <span class="hints">E</span> </div> <div data-key="68" class="key" data-note="E"> <span class="hints">D</span> </div> <div data-key="70" class="key" data-note="F"> <span class="hints">F</span> </div> <div data-key="84" class="key sharp" data-note="F#"> <span class="hints">T</span> </div> <div data-key="71" class="key" data-note="G"> <span class="hints">G</span> </div> <div data-key="89" class="key sharp" data-note="G#"> <span class="hints">Y</span> </div> <div data-key="72" class="key" data-note="A"> <span class="hints">H</span> </div> <div data-key="85" class="key sharp" data-note="A#"> <span class="hints">U</span> </div> <div data-key="74" class="key" data-note="B"> <span class="hints">J</span> </div> <div data-key="75" class="key" data-note="C"> <span class="hints">K</span> </div> <div data-key="79" class="key sharp" data-note="C#"> <span class="hints">O</span> </div> <div data-key="76" class="key" data-note="D"> <span class="hints">L</span> </div> <div data-key="80" class="key sharp" data-note="D#"> <span class="hints">P</span> </div> <div data-key="186" class="key" data-note="E"> <span class="hints">;</span> </div> </div> <audio data-key="65" src="https://carolinegabriel.com/demo/js-keyboard/sounds/040.wav"></audio> <audio data-key="87" src="https://carolinegabriel.com/demo/js-keyboard/sounds/041.wav"></audio> <audio data-key="83" src="https://carolinegabriel.com/demo/js-keyboard/sounds/042.wav"></audio> <audio data-key="69" src="https://carolinegabriel.com/demo/js-keyboard/sounds/043.wav"></audio> <audio data-key="68" src="https://carolinegabriel.com/demo/js-keyboard/sounds/044.wav"></audio> <audio data-key="70" src="https://carolinegabriel.com/demo/js-keyboard/sounds/045.wav"></audio> <audio data-key="84" src="https://carolinegabriel.com/demo/js-keyboard/sounds/046.wav"></audio> <audio data-key="71" src="https://carolinegabriel.com/demo/js-keyboard/sounds/047.wav"></audio> <audio data-key="89" src="https://carolinegabriel.com/demo/js-keyboard/sounds/048.wav"></audio> <audio data-key="72" src="https://carolinegabriel.com/demo/js-keyboard/sounds/049.wav"></audio> <audio data-key="85" src="https://carolinegabriel.com/demo/js-keyboard/sounds/050.wav"></audio> <audio data-key="74" src="https://carolinegabriel.com/demo/js-keyboard/sounds/051.wav"></audio> <audio data-key="75" src="https://carolinegabriel.com/demo/js-keyboard/sounds/052.wav"></audio> <audio data-key="79" src="https://carolinegabriel.com/demo/js-keyboard/sounds/053.wav"></audio> <audio data-key="76" src="https://carolinegabriel.com/demo/js-keyboard/sounds/054.wav"></audio> <audio data-key="80" src="https://carolinegabriel.com/demo/js-keyboard/sounds/055.wav"></audio> <audio data-key="186" src="https://carolinegabriel.com/demo/js-keyboard/sounds/056.wav"></audio> </section> </section> <video playsinline autoplay muted loop id="bgvid" poster="http://carolinegabriel.com/demo/js-keyboard/video/bg.jpg"> <source src="https://carolinegabriel.com/demo/js-keyboard/video/bg.mp4" type="video/mp4"> </video>
2. Now, add the following CSS code to your project. You can modify the CSS code to change the piano’s appearance, such as background color, font, or key size. Adjust the styles in the CSS section to match your website’s design.
html { background: #000; font-family: 'Noto Serif', serif; -webkit-font-smoothing: antialiased; text-align: center; } video#bgvid { position: fixed; top: 50%; left: 50%; min-width: 100%; min-height: 100%; width: auto; height: auto; z-index: -100; transform: translateX(-50%) translateY(-50%); background-size: cover; } header { position: relative; margin: 30px 0; } header:after { content: ''; width: 460px; height: 15px; background: url(images/intro-div.svg) no-repeat center; display: inline-block; text-align: center; background-size: 70%; } #wrap h1 { color: #fff; font-size: 50px; font-weight: 400; letter-spacing: 0.18em; text-transform: uppercase; margin: 0; } #wrap h2 { color: #fff; font-size: 24px; font-style: italic; font-weight: 400; margin: 0 0 30px; } .nowplaying { font-size: 120px; line-height: 1; color: #eee; text-shadow: 0 0 5rem #028ae9; transition: all .07s ease; min-height: 120px; } .keys { display: block; width: 100%; height: 350px; max-width: 880px; position: relative; margin: 40px auto 0; cursor: none; } .key { position: relative; border: 4px solid black; border-radius: .5rem; transition: all .07s ease; display: block; box-sizing: border-box; z-index: 2; } .key:not(.sharp) { float: left; width: 10%; height: 100%; background: rgba(255, 255, 255, .8); } .key.sharp { position: absolute; width: 6%; height: 60%; background: #000; color: #eee; top: 0; z-index: 3; } .key[data-key="87"] { left: 7%; } .key[data-key="69"] { left: 17%; } .key[data-key="84"] { left: 37%; } .key[data-key="89"] { left: 47%; } .key[data-key="85"] { left: 57%; } .key[data-key="79"] { left: 77%; } .key[data-key="80"] { left: 87%; } .playing { transform: scale(.95); border-color: #028ae9; box-shadow: 0 0 1rem #028ae9; } .hints { display: block; width: 100%; opacity: 0; position: absolute; bottom: 7px; transition: opacity .3s ease-out; font-size: 20px; } .keys:hover .hints { opacity: 1; }
3. Finally, add the following JavaScript code to your project. It handles the piano keyboard functionality, keypress events, and audio playback.
const keys = document.querySelectorAll(".key"), note = document.querySelector(".nowplaying"), hints = document.querySelectorAll(".hints"); function playNote(e) { const audio = document.querySelector(`audio[data-key="${e.keyCode}"]`), key = document.querySelector(`.key[data-key="${e.keyCode}"]`); if (!key) return; const keyNote = key.getAttribute("data-note"); key.classList.add("playing"); note.innerHTML = keyNote; audio.currentTime = 0; audio.play(); } function removeTransition(e) { if (e.propertyName !== "transform") return; this.classList.remove("playing"); } function hintsOn(e, index) { e.setAttribute("style", "transition-delay:" + index * 50 + "ms"); } hints.forEach(hintsOn); keys.forEach(key => key.addEventListener("transitionend", removeTransition)); window.addEventListener("keydown", playNote);
That’s all! hopefully, you have successfully created a JavaScript Piano Keyboard App. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
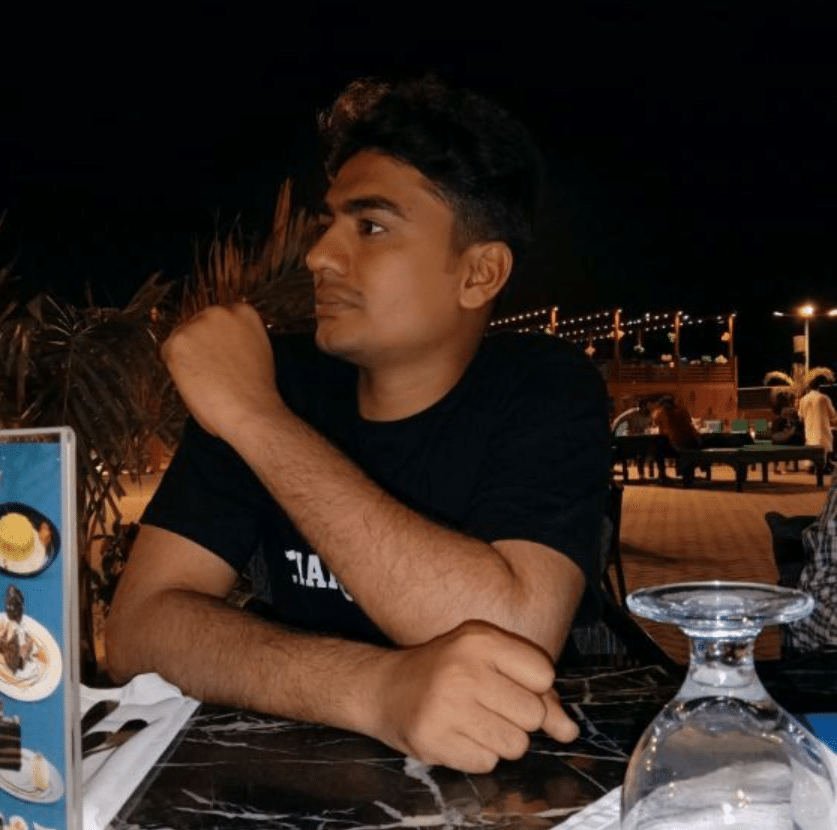
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.