This JavaScript code snippet helps you to create a web-based tool for temperature converter to easily convert Fahrenheit to Celsius. It comes with a basic interface where users can select Celsius or Fahrenheit, input a temperature, and instantly convert it. It’s helpful for easy temperature unit conversion on websites.
You can integrate this code into your JavaScript programs within web applications, like weather apps or cooking websites, to provide a user-friendly temperature conversion feature.
How to Create Fahrenheit To Celsius Converter Using JavaScript
1. First, copy the following HTML code into your web page’s HTML file. This code creates a user interface with a dropdown menu to select the unit (Celsius or Fahrenheit), an input field to enter the temperature, and a paragraph to display the converted result.
<form> <select id="temperature" onchange="convertTemperature()"> <option value="celsius">Celsius</option> <option value="fahrenheit">Fahrenheit</option> </select> <input id="enteredTemp" onkeyup="convertTemperature()" type="number" /> <p id="result"></p> </form>
2. If you want to style the interface, you can modify the following CSS code. It’s optional, but you can adjust fonts, colors, and layout to match your website’s design.
@import url(https://fonts.googleapis.com/css?family=Passion+One); @import url(https://fonts.googleapis.com/css?family=Cherry+Swash); h2{ font-family: 'Cherry Swash'; font-size: 20px; color: #00A94F; } p { font-family: 'Passion One'; } #result { text-align: left; font-size: 18px; } input { width: 50px; font-family: 'Passion One'; border-top: none; border-left: none; border-right: none; border-bottom: 1px solid gray; outline: none; } /* Hides arrows in iput */ /* Chrome, Safari, Edge, Opera */ input::-webkit-outer-spin-button, input::-webkit-inner-spin-button { -webkit-appearance: none; margin: 0; } /* Firefox */ input[type=number] { -moz-appearance:textfield; } select { font-family: 'Passion One'; color: #00A94F; border: none; outline: none; }
3. Finally, copy the JavaScript code into your JavaScript file or script tag within your HTML. This code defines a JavaScript function called “convertTemperature.” It retrieves the selected unit and entered temperature, performs the conversion, and displays the result in the p
element.
function convertTemperature () { let selectedTemp = document.getElementById("temperature").value; let enteredTemp = document.getElementById("enteredTemp").value; // if no value entered clears the text if (enteredTemp == "") { document.getElementById("result").innerHTML = null; return; } // if Celsius selected if (selectedTemp == "celsius") { let fahr = ((9 * enteredTemp) / 5) +32; document.getElementById("result").innerHTML = fahr.toFixed(2) + " °F"; return; } // if Fahrenheit selected if (selectedTemp == "fahrenheit") { let cel = ((enteredTemp - 32) / 9) * 5; document.getElementById("result").innerHTML = cel.toFixed(2) + " °C"; } }
You can further customize the code to fit your specific needs. For example, you can change the styling, add error handling, or integrate it into a larger application.
That’s all! hopefully, you have successfully created Fahrenheit to Celsius Converter using this JavaScript Code. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
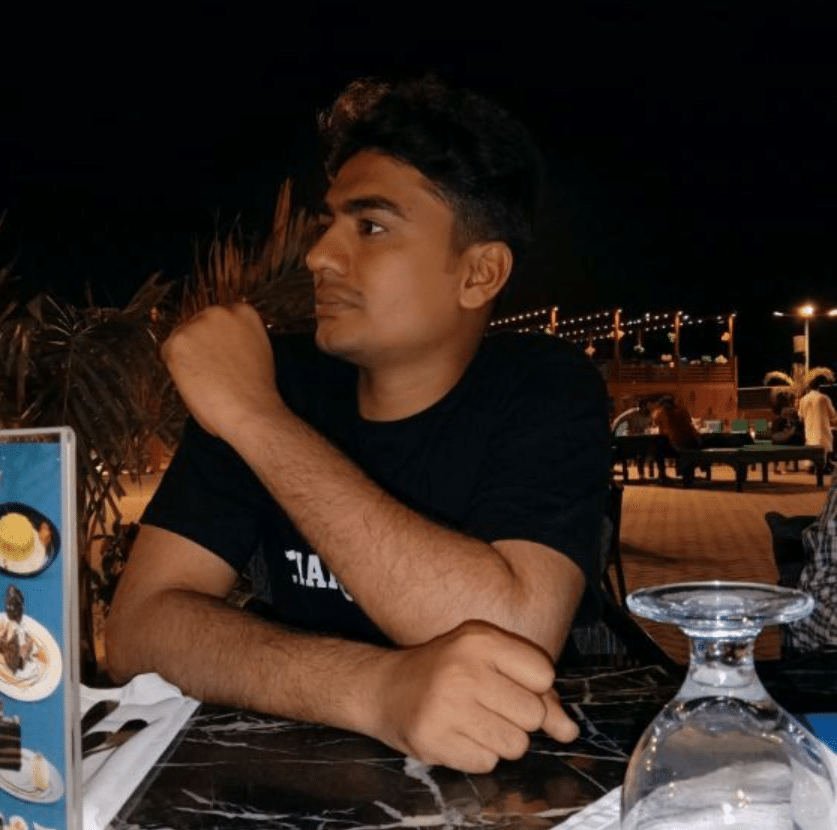
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.