This JavaScript code helps you to convert an image to base64 format. It operates by allowing you to select a file using an input field, and upon selection, it transforms the file into a Base64 data URI. This code can be helpful for quickly converting files into a format that’s easily shareable and embeddable in web applications.
You can use this code on your website to enable users to quickly convert image and text files to Base64 format. This can be beneficial for reducing the number of HTTP requests, making your site load faster, and simplifying data sharing.
It also helps in embedding images and files directly in web pages without separate file downloads.
How to Convert Image To Base64 Using JavaScript
1. First, copy the following HTML code into your webpage. This code includes the necessary structure for file input and display of the Base64 data. Customize the HTML structure to fit your webpage layout, such as changing the button labels or adding additional elements.
<div class='kepala-base'> IMAGE<span> BASE64 CONVERTER</span></div> <div class='wrapper'> <br /> <input type="file" id="files" class="inputFile" name="files[]" /> </div> <!-- lightbox --> <div class="overlay"> <a href="#" class="close_overlay">x</a> <div class="output"></div> <br /> </div>
2. Now, copy the CSS code to style the interface elements as desired. Customize the CSS to match your website’s design.
/* = body ----------------------------*/ html,body{ background:#fff; position:relative; height:100%; margin:40px; font-family: Arial, "sans-serief"; } span{ color:#fff; } .kepala-base{ width:95%; background:#555; border:2px solid #555; color:#fff; padding:20px 30px; text-align:center; font-size:20px; } .wrapper{ background:#454851; border:2px solid #555; border-radius: 0px 0 3px 3px; padding:30px 30px; display: block; margin: 0; width: 95%; height:200px; text-align: center; } /* = input file ----------------------------*/ .inputFile { background:transparent; color: transparent; outline:none; cursor:pointer; display:block; margin:0 auto; } .inputFile::-webkit-file-upload-button { visibility: hidden; } .inputFile::before { content: 'Select File'; margin: 10px; color: #000; display: inline-block; background: #fff; padding: 5px; width: 100px; height: 48px; line-height: 40px; text-align: center; text-decoration:none; outline: none; white-space: nowrap; -webkit-user-select: none; cursor: pointer; font-family: 'Oswald', 'sans-serif'; font-weight: 300; font-size: 20px; border-radius: 2px; transition:all 0.2s ease; } .inputFile:hover::before { color: #eee; transition:all 0.2s ease; } .inputFile:active { outline: 0; } .inputFile:active::before { color: #FFF; } input:focus,input:hover{background:#454851} .btn{ margin:5px; color: #FFF; display: inline-block; background: #757D75; padding: 10px 14px; text-align: center; text-decoration:none; outline: none; white-space: nowrap; cursor: pointer; font-family: 'Oswald', 'sans-serif'; font-weight: 300; font-size: 20px; border-radius: 2px; transition:all 0.2s ease; } .btn:hover{ background: #4A6266; color: #fafafa; transition:all 0.2s ease; } /* = lightbox ----------------------------*/ .overlay{ position: fixed; top: 0; left: 0; padding: 0; margin: 0; opacity: 0; visibility: hidden; width: 100%; height:199%; background:#757D75; -webkit-transition: all 0.5s ease; transition: all 0.5s ease; } .close_overlay { position: absolute; top: 0; right: 0; margin: 0; padding: 0; text-align: center; font-family: sans-serif; text-decoration: none; font-size: 40px; width: 50px; height: 50px; line-height: 45px; background:#F46B63; color:#fafafa; transition: all 0.5s ease; } .close_overlay:hover{ background: #4A6266; color: #fafafa; transition:all 0.5s ease; } .show{ background:#6C7767; opacity: 1; visibility: visible; z-index: 999999999; } .output { background:#8BA987; position: fixed; top: 0; left: 0; right: 0; margin: 5% auto; padding: 1em; width: 600px; height: 500px; overflow:auto; } .output ul { width: 100%; list-style: none; margin: 0; padding: 3px; display: block; color: #000; font-size: 13px; } .output ul b{ color: #000; } .textarea { overflow: auto; width: 100%; height: 200px; margin: auto; margin-bottom:16px; display: block; border: 1px solid #ecf0f1; background: #ecf0f1; color: #7f8c8d; font-size:13px; font-family:monospace,sans-serif; word-break: break-all; word-wrap: break-word; white-space: pre; white-space: -moz-pre-wrap; white-space: pre-wrap; white-space: pre\9; } .thumb { display: block; width: 50%; height: auto; margin: 10px auto; box-shadow: 0 6px 6px -6px #000; }
3. Finally, add the JavaScript code to your webpage. This code handles the file selection, conversion, and display of the Base64 data.
var img2data = (function() { 'use strict'; return { // this.qS(foo) qS: function(el) { return document.querySelector(el); }, run: function() { this.convert(); }, convert: function() { // vars var fls = this.qS('#files'), output = this.qS('.output'), overlay = this.qS('.overlay'), close_overlay = this.qS('.close_overlay'); fls.addEventListener('change', function(e) { var file = fls.files[0], txtType = /text.*/, // all text files imgType = /image.*/, // all image files fR = new FileReader(); // fileReader start fR.onload = function(e) { // if text if (file.type.match(txtType)) { var rS = fR.result, // template render = '<a class="btn" href="'+rS+'" target="blank">Output</a><ul>'+ '<li><b>Name: </b> '+file.name+'</li>'+ '<li><b>Size: </b> '+file.size+'</li>'+ '<li><b>Type: </b> '+file.type+'</li>'+ '<li><b>Data uri: </b></li>'+ '</ul>'+ '<pre class="textarea">'+rS+'</pre>'; output.innerHTML = render; // if image } else if(file.type.match(imgType)) { var rS2 = fR.result, // template tmpl = '<a class="btn" href="'+rS2+'" target="blank">Output</a>'+ '<img class="thumb" src="'+rS2+'" alt="'+file.name+'"><ul>'+ '<li><b>Name: </b> '+file.name+'</li>'+ '<li><b>Size: </b> '+file.size+'</li>'+ '<li><b>Type: </b> '+file.type+'</li>'+ '<li><b>Data uri: </b></li>'+ '</ul>'+ '<pre class="textarea">'+rS2+'</pre>'; output.innerHTML = tmpl; // if not support }else{ output.innerHTML = '<h1>Maaf file yang anda upload tidak mendukung</h1>'; } }; // on loaded add events fR.onloadend = function(e) { overlay.classList.add('show'); // add class close_overlay.onclick = function(){ overlay.classList.remove('show'); // remove class fls.value = ''; // remove files }; }; // convert to data uri fR.readAsDataURL(file); }); } }; })(); img2data.run();
That’s all! hopefully, you have successfully created a functionality to convert image to base64 using JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
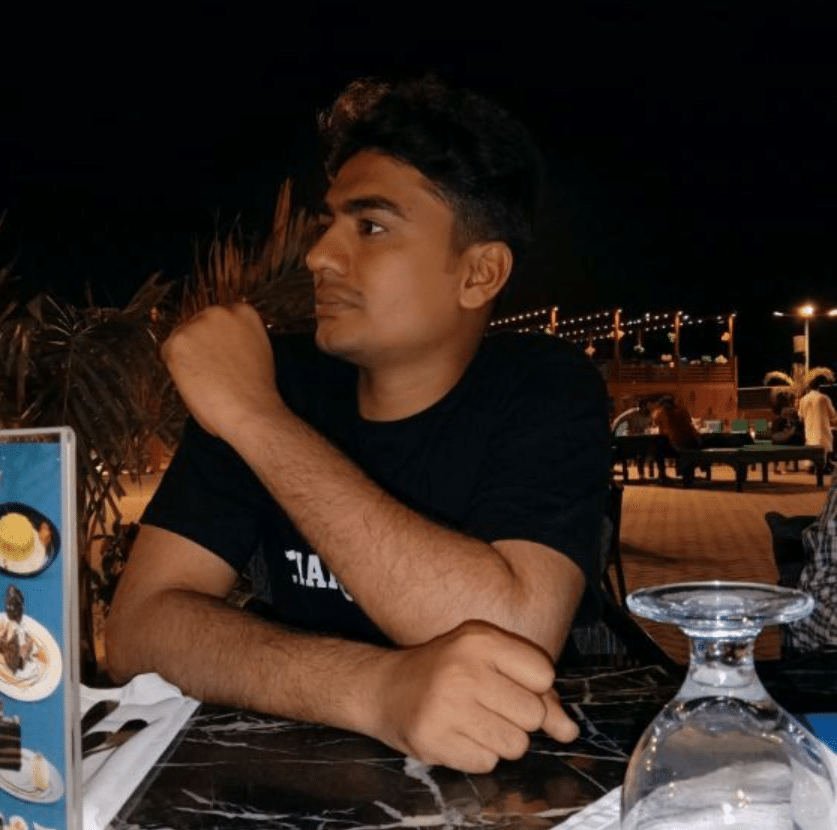
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.