This Vanilla JavaScript code snippet helps you to create a password visibility eye icon toggle button to show/hide passwords. It gets the password input field and changes its type from password to text to show the entered password. The snippet uses Font Awesome eye icon inside the password input field and Bootstrap CSS for basic input design. However, you can integrate it with Bootstrap 4/5 or with your custom-designed login/signup form.
How to Create Password Eye Icon Visibility JavaScript
First of all, load Bootstrap (optional) and Font Awesome CSS into the head tag of your HTML document.
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" integrity="sha384-BVYiiSIFeK1dGmJRAkycuHAHRg32OmUcww7on3RYdg4Va+PmSTsz/K68vbdEjh4u" crossorigin="anonymous"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
After that, create the HTML password input with a unique id “passInput”. Create a span element just after the input with an id “passBtn” and place Font Awesome eye icon inside it.
<div class="form-wrapper"> <form id="form_id" class="form-class"> <p class="input-group"> <input id="passInput" class="form-control" placeholder="Your Password" name="password" type="password" size="30" aria-required="false"> <span class="input-group-addon" role="button" title="veiw password" id="passBtn"> <i class="fa fa-eye fa-fw" aria-hidden="true"></i> </span> </p> </form> </div>
If you are using Bootstrap, you don’t need any additional CSS styles.
.form-wrapper { margin: 10rem auto; max-width: 30rem; }
Finally, add the following JavaScript function to toggle the password visibility on the click event.
const PassBtn = document.querySelector('#passBtn'); PassBtn.addEventListener('click', () => { const input = document.querySelector('#passInput'); input.getAttribute('type') === 'password' ? input.setAttribute('type', 'text') : input.setAttribute('type', 'password'); if (input.getAttribute('type') === 'text'){ PassBtn.innerHTML = '<i class="fa fa-eye-slash"></i>'; } else{ PassBtn.innerHTML = '<i class="fa fa-eye fa-fw" aria-hidden="true"></i>'; } });
That’s all! hopefully, you have successfully created a password visibility toggle using JavaScript. If you have any questions or suggestions, feel free to comment below.
Connect with us on social media:
- https://www.intensedebate.com/profiles/codehimcom
- https://community.atlassian.com/t5/user/viewprofilepage/user-id/5256177
- https://trello.com/u/codehim
- https://kit.co/codehim
Similar Code Snippets:
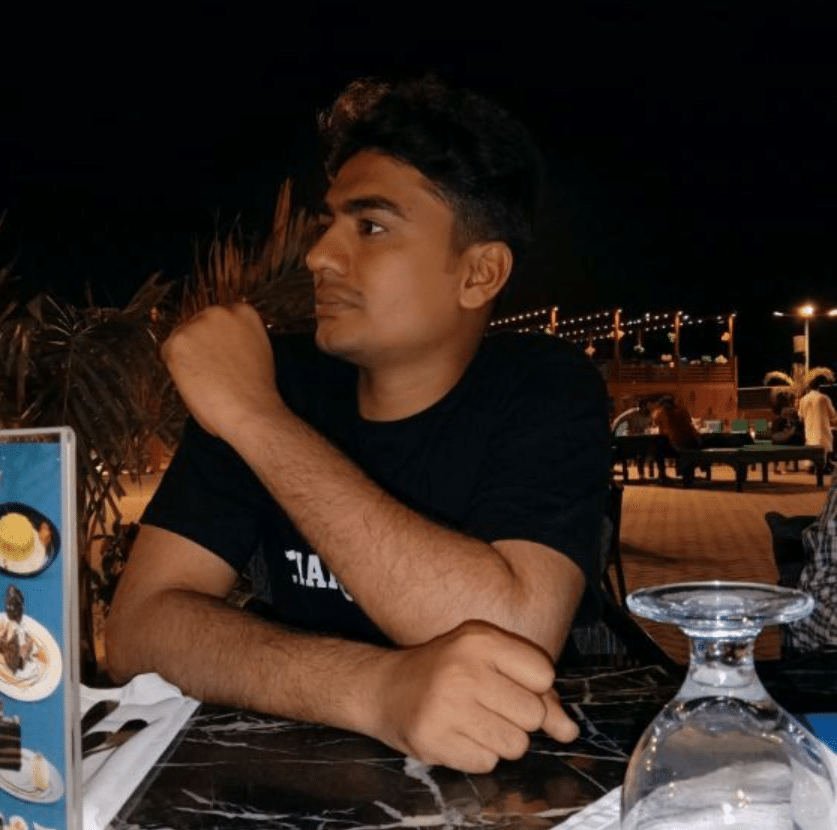
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.