This lightweight Vanilla JavaScript code snippet helps you to create an easy sliding drawer menu navigation. It comes with brand logo and navigation links arranged in a side nav that smoothly open/close with a button. Besides this, a dim background overlay shows over the main content when nav opens. The menu also close when a user clicks outside the menu drawer.
How to Create Easy Sliding Drawer In Vanilla JavaScript
1. First of all, create the HTML structure as follows:
<div class="overlay"></div> <nav class="nav"> <div class="toggle"> <span class="toggler">»</span> </div> <div class="logo"> <a href="#">CodeHim</a> </div> <ul> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Projects</a></li> <li><a href="#">Blog</a></li> <li><a href="#">Contact</a></li> </ul> </nav> <main> <div class="container"> <div class="content"> <h1>A Simple JavaScript Drawer</h1> <div class="post"> <p class="lead"> This page demonstrates the easy sliding drawer. Use the menu open button (shown on the top right corner) to slide the menu in. You can close the menu drawer by clicking the same button or dim overlay. </p> </div> </div> </div> </main>
2. After that, add the following CSS styles to your project:
*, *::after, *::before { padding: 0; margin: 0; box-sizing: border-box; } body { background-color: #e8e8e8; } .overlay { width: 100%; height: 100%; position: absolute; left: 0; top: 0; background-color: rgba(0,0,0,.3); z-index: 90; opacity: 0; visibility: hidden; transition: all 200ms ease-in; } .nav { width: 270px; height: 100vh; background-color: #262626; left: -270px; top: 0; position: fixed; padding: 20px 0; box-shadow: 0 2px 4px rgba(0,0,0,.6); transition: all 200ms ease-in-out; z-index: 99; } .toggle { position: absolute; left: 100%; background-color: #262626; width: 40px; height: 40px; color: #fff; display: flex; align-items: center; justify-content: center; font-size: 20px; border-top-right-radius: 30px; border-bottom-right-radius: 30px; cursor: default; } .toggle span { display: block; margin-top: -5px; } .logo { text-align: center; margin-bottom: 30px; } .logo a{ text-decoration: none; color: #888; font-size: 30px; text-shadow: 0 1px 3px rgba(0,0,0,.6); font-family: Arial, Helvetica, sans-serif; } .nav ul li { display: block; list-style: none; } .nav ul li a { padding: 10px 20px; display: block; color: #f6f6f6; font-family: Arial, Helvetica, sans-serif; font-size: 17px; text-decoration: none; transition: all 200ms ease; } .nav ul li a:hover { background-color: #444; } /* Show Nav */ .show-nav .nav { left: 0; } .show-nav .overlay { opacity: 1; visibility: visible; } /* Styling the content */ .container { max-width: 1000px; margin: 0 auto; } .content { background-color: #fff; margin-top: 100px; padding: 20px; font-family: Arial, Helvetica, sans-serif; box-shadow: 0 1px 2px rgba(0,0,0,.1) } .content h1 { text-align: center; margin-bottom: 30px; font-size: 35px; color: #333; } .content .lead { color: #333; font-size: 18px; font-weight: 600; text-align: center; line-height: 1.7; }
3. Finally, add the following JavaScript code for sliding drawer and done.
let toggler = document.querySelector(".toggler"); window.addEventListener("click", event => { if(event.target.className == "toggler" || event.target.className == "toggle") { document.body.classList.toggle("show-nav"); } else if (event.target.className == "overlay") { document.body.classList.remove("show-nav"); } // Change Toggler Icon if(document.body.className == "show-nav") { toggler.innerHTML = "«"; } else { toggler.innerHTML = "»"; } });
That’s all! hopefully, you have successfully integrated this sliding drawer code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
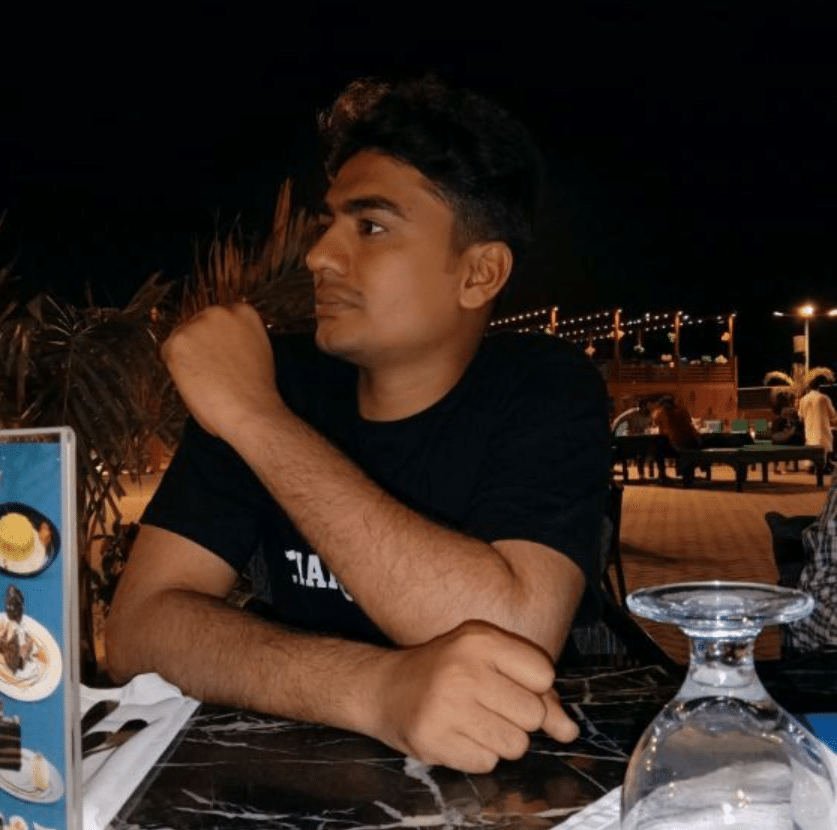
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.