The Poptrox is a lightweight, AJAX-ready, responsive, and flat design jQuery lightbox with caption. It helps you to create a gallery to show images, videos (YouTube, Vimeo, Wistia, Brightcove), Soundcloud tracks, and iframe in a popup lightbox. It can be highly customized with CSS & its available options.
How to Create a Lightbox with Caption using jQuery
1. Load the jQuery and poptrox
‘s JavaScript file into your HTML document.
<!-- jQuery --> <script src="https://code.jquery.com/jquery-1.11.1.min.js"></script> <!-- Poptrox JS --> <script src="js/jquery.poptrox.min.js"></script>
2. Create the HTML structure for a basic gallery and put your images in it.
<div id="gallery"> <a href="images/1.jpg"><img src="images/1_thumb.jpg" alt="" title="Just an image (#1)" /></a> <a href="images/2.jpg"><img src="images/2_thumb.jpg" alt="" title="Just an image (#2)" /></a> <a href="images/3.jpg"><img src="images/3_thumb.jpg" alt="" title="Just an image (#3)" /></a> </div>
3. If you want to add videos & iframe contents, similarly use the following structure.
<div id="gallery"> <a href="http://youtu.be/loGm3vT8EAQ" data-poptrox="youtube,800x480"><img src="images/youtube.png" alt="" title="A YouTube Video" /></a> <a href="http://vimeo.com/22439234" data-poptrox="vimeo,800x480"><img src="images/vimeo.png" alt="" title="A Vimeo Video" /></a> <a href="http://api.soundcloud.com/tracks/93549370" data-poptrox="soundcloud"><img src="images/soundcloud.png" alt="" title="A Soundcloud Track" /></a> <a href="http://bcove.me/qly3wjdw" data-poptrox="bcove,636x360"><img src="images/brightcove.png" alt="" title="A Brightcove Video" /></a> <a href="http://fast.wistia.net/embed/iframe/fe8t32e27x" data-poptrox="wistia,800x480"><img src="images/wistia.png" alt="" title="A Wistia Video" /></a> <a href="iframe.html" data-poptrox="iframe,600x400"><img src="images/iframe.png" alt="" title="An IFRAME" /></a> </div>
4. Finally, initialize the plugin in the jQuery document ready function.
$(document).ready(function(){ $('#gallery').poptrox(); });
5. The popup lightbox doesn’t require any CSS style, it will generate styles dynamically. However, if you want to style your gallery, then the basic CSS styles for gallery layout.
#wrapper { max-width: 600px; margin: 0 auto; text-align: center; } #gallery { overflow: hidden; } #gallery a { display: block; float: left; } #gallery a img { display: block; border: 0; }
Advanced Configuration Options
The following are advanced configuration options to create/customize “poptrox lightbox”.
$('#gallery').poptrox({ preload: false, // If true, preload fullsize images in // the background baseZIndex: 1000, // Base Z-Index fadeSpeed: 300, // Global fade speed overlayColor: '#000000', // Overlay color overlayOpacity: 0.6, // Overlay opacity windowMargin: 50, // Window margin size (in pixels; only comes into // play when an image is larger than the viewport) windowHeightPad: 0, // Window height pad selector: 'a', // Anchor tag selector caption: null, // Caption settings (see docs) popupSpeed: 300, // Popup (resize) speed popupWidth: 200, // Popup width popupHeight: 100, // Popup height popupIsFixed: false, // If true, popup won't resize to fit images useBodyOverflow: true, // If true, the BODY tag is set to overflow: hidden // when the popup is visible usePopupEasyClose: true, // If true, popup can be closed by clicking on // it anywhere usePopupForceClose: false, // If true, popup can be closed even while content // is loading usePopupLoader: true, // If true, show the popup loader usePopupCloser: true, // If true, show the popup closer button/link usePopupCaption: false, // If true, show the popup image caption usePopupNav: false, // If true, show (and use) popup navigation usePopupDefaultStyling: true, // If true, default popup styling will be applied // (background color, text color, etc) popupBackgroundColor: '#FFFFFF', // (Default Style) Popup background color (when // usePopupStyling = true) popupTextColor: '#000000', // (Default Style) Popup text color (when // usePopupStyling = true) popupLoaderTextSize: '2em', // (Default Style) Popup loader text size popupCloserBackgroundColor: '#000000', // (Default Style) Popup closer background color // (when usePopupStyling = true) popupCloserTextColor: '#FFFFFF', // (Default Style) Popup closer text color (when // usePopupStyling = true) popupCloserTextSize: '20px', // (Default Style) Popup closer text size popupPadding: 10, // (Default Style) Popup padding (when // usePopupStyling = true) popupCaptionHeight: 60, // (Default Style) Popup height of caption area popupCaptionTextSize: null, // (Default Style) Popup caption text size popupBlankCaptionText: '(untitled)', // Applied to images that don't have captions // (when captions are enabled) popupCloserText: '×', // Popup closer text popupLoaderText: '••', // Popup loader text popupClass: 'poptrox-popup',// Popup class popupSelector: null, // (Advanced) Popup selector (use this if you // want to replace the built-in popup) popupLoaderSelector: '.loader', // (Advanced) Popup Loader selector popupCloserSelector: '.closer', // (Advanced) Popup Closer selector popupCaptionSelector: '.caption', // (Advanced) Popup Caption selector popupNavPreviousSelector: '.nav-previous',// (Advanced) Popup Nav Previous selector popupNavNextSelector: '.nav-next', // (Advanced) Popup Nav Next selector onPopupClose: null, // Called when popup closes onPopupOpen: null // Called when popup opens });
That’s all! hopefully, you have successfully created a lightbox with a caption. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
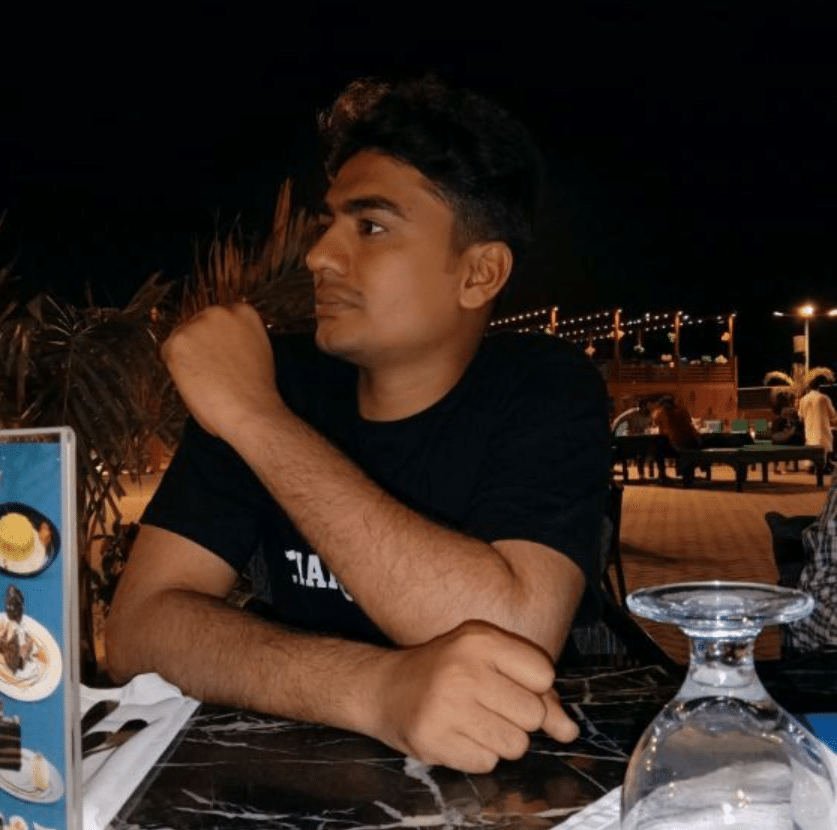
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.