This HTML code snippet helps you to create a show more / show less feature for content containers. It limits content visibility initially. Clicking “Show more” expands the content, and “Show less” collapses it. This functionality helps manage lengthy content visibility.
You can use this code on websites with long content, like blogs or product descriptions. The benefit is offering users control over content visibility, and enhancing readability.
How to Create Show More / Show Less HTML Code
1. Insert the HTML structure for your content containers. Each container should have a container
class wrapping the content inside a content
class.
<div class="container"> <div class="content"> <p> Content for container 1. orem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum </p> </div> </div> <div class="container"> <div class="content"> <p> Content for container 2. orem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum </p> </div> </div>
2. Style your containers using CSS. Adjust the max-height
property in the .content
class to determine the initial height of the content to display. Add styling for the “Show more” button, defining its appearance and transitions.
.container { max-width: 600px; margin: 20px auto; font-family: Arial, sans-serif; } .content { max-height: 100px; /* Adjust the initial max-height as needed */ overflow: hidden; transition: max-height 0.3s ease; /* Add smooth transition effect */ } .show-more { cursor: pointer; color: blue; text-decoration: none; background: linear-gradient(to bottom, #3a7bd5, #3a6073); /* Gradient background color */
3. Finally, place the JavaScript code inside a <script>
tag or in an external JavaScript file. The code uses the document.querySelectorAll
method to select all elements with the content
class. For each content container, a “Show more” button is created and appended before the content.
Event listeners are attached to these buttons. When clicked, they toggle the max-height
property of the content to reveal or hide the entire content.
document.addEventListener('DOMContentLoaded', function () { const contentContainers = document.querySelectorAll('.content'); contentContainers.forEach((content, index) => { const showMoreButton = document.createElement('div'); showMoreButton.classList.add('show-more'); showMoreButton.innerHTML = '<span>+</span> Show more'; content.parentNode.insertBefore(showMoreButton, content.nextSibling); showMoreButton.addEventListener('click', function () { if (content.style.maxHeight) { content.style.maxHeight = null; showMoreButton.innerHTML = '<span>+</span> Show more'; } else { content.style.maxHeight = content.scrollHeight + 'px'; showMoreButton.innerHTML = '<span>-</span> Show less'; } }); }); });
That’s all! hopefully, you have successfully integrated this Show More/Show Less HTML code into your project. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
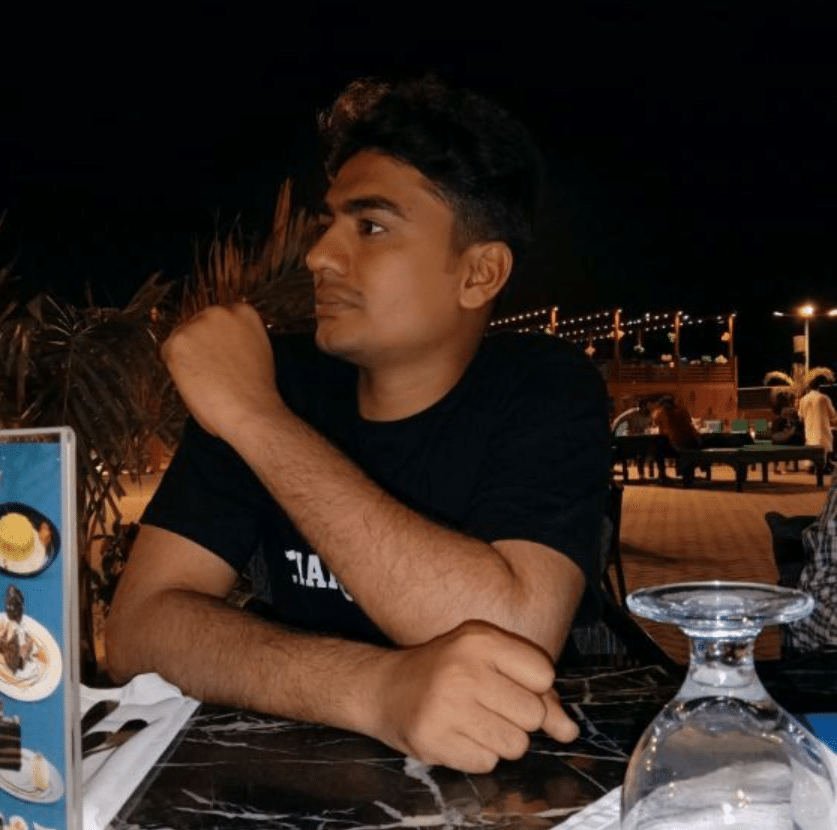
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.