This JavaScript code creates 25 seconds of confetti animation on a single click. It utilizes tsParticles for confetti effects. The “Party” button triggers the animation, adding excitement to your webpage.
You can use this code on your website’s landing page to add interactive elements. It enhances user engagement with fun and dynamic visual effects. The confetti animation adds a festive touch to celebrations or special events on your site.
How to Create 25 Seconds Of Confetti Animation in JavaScript
1. First, ensure you have the necessary HTML structure in your webpage. Include a container with a button to trigger the confetti animation.
<div id="layout"> <div></div> <div id="sublayout"> <div id="button-container"> <button type="button" id="party">Party</button> </div> </div> <div></div> </div>
2. Apply CSS styles to ensure the layout and button appear as desired. Customize according to your website’s design.
body { cursor: pointer; background: #000 !important; min-height: 720px; position: relative; } #layout { position: absolute; height: 100%; width: 100%; top: 0; left: 0; align-items: center; display: flex; } #layout > div { flex: 2; } #sublayout { display: flex; flex: 1 !important; flex-direction: row; align-items: center; } #button-container { flex: 1; text-align: center; } #party { width: 100px; height: 100px; font-size: 30px; border-radius: 50px; border: none; background: transparent; color: #fff; box-shadow: 4px 4px 5px #fff, -4px -4px 5px #fff, inset 4px 4px 5px #ababab; } #party:active { box-shadow: 4px 4px 5px #fff, -4px -4px 5px #fff, inset 4px 4px 5px #000; }
3. Now, add the required scripts for the confetti animation. These scripts include the tsParticles library and the confetti preset.
<script src='https://cdn.jsdelivr.net/npm/[email protected]/tsparticles.min.js'></script> <script src='https://cdn.jsdelivr.net/npm/[email protected]/tsparticles.preset.confetti.min.js'></script>
4. Finally, implement the JavaScript code to enable the confetti animation. This code utilizes the tsParticles library for easy confetti effects.
//I do not code in vanilla javascript, but loved this pen and played around with it, https://codepen.io/wakana-k/pen/qBLMVOG - go visit Wakana for some truly awesome pens! //Original pen here with many thanks to: https://codepen.io/matteobruni/pen/wvprNwP //one click anywhere for 25 seconds of confetti //uses tsParticles for confetti loadConfettiPreset(tsParticles); let confettiContainer; const partyBtn = document.getElementById("party"); tsParticles .load("tsparticles", { particles: { shape: { type: "character", character: { value: ["*"] },move: { direction: "top"}, } }, preset: "confetti", emitters: [] }) .then((container) => (confettiContainer = container)); layout.addEventListener("click", () => { confettiContainer.addEmitter({ size: { width: 0, height: 0 }, startCount: 340, position: { "x": 50, "y": 50}, rate: { delay: 0, quantity: 50 }, life: { duration: 5, count: 5 } }); });
Once you’ve implemented the code, test the functionality by clicking the “Party” button. Enjoy the vibrant confetti animation on your website!
That’s it! You’ve successfully added a dynamic confetti animation to your webpage using JavaScript and tsParticles. Customize the code and styles further to suit your website’s theme and preferences.
Similar Code Snippets:
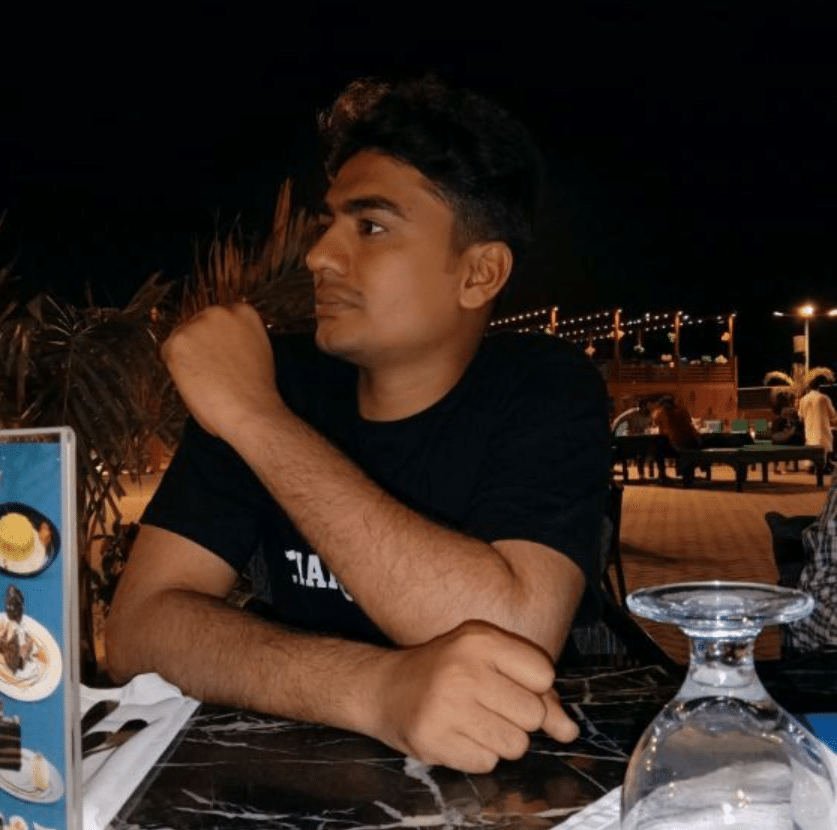
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.