This lightweight JavaScript code snippet helps you to create an animated background for website with HTML5 canvas. It uses requestAnimationFrame function to generate random bubbles with bounce animation to make an attractive background.
How to Create Animated Website Background with HTML5
1. First of all, create the HTML structure as follows:
<div class="container py-5 text-white"> <div class="row justify-content-center text-center"> <div class="col-12"> <h1 class="pb-3 h5">Number counter animation <br> in pure JavaScript <br> (requestAnimationFrame) </h1> </div> <div class="col-md-3"> <span id="count1" class="display-4"></span> </div> <div class="col-md-3"> <span id="count2" class="display-4"></span> </div> <div class="col-md-3"> <span id="count3" class="display-4"></span> </div> <div class="col-md-3"> <span id="count4" class="display-4"></span> </div> </div> </div>
2. After that, add the following CSS styles to your project:
/* I'm too lazy to write CSS 🌠... other than that Bootstrap has nothing to do with this example. */ body { background-color: #000; } main{ background: #8E2DE2; /* fallback for old browsers */ background: -webkit-linear-gradient(to right, #4A00E0, #8E2DE2); /* Chrome 10-25, Safari 5.1-6 */ background: linear-gradient(to right, #4A00E0, #8E2DE2); /* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */ }
3. Finally, add the following JavaScript code and done.
//#region - start of - number counter animation const counterAnim = (qSelector, start = 0, end, duration = 1000) => { const target = document.querySelector(qSelector); let startTimestamp = null; const step = (timestamp) => { if (!startTimestamp) startTimestamp = timestamp; const progress = Math.min((timestamp - startTimestamp) / duration, 1); target.innerText = Math.floor(progress * (end - start) + start); if (progress < 1) { window.requestAnimationFrame(step); } }; window.requestAnimationFrame(step); }; //#endregion - end of - number counter animation document.addEventListener("DOMContentLoaded", () => { counterAnim("#count1", 10, 300, 1000); counterAnim("#count2", 5000, 250, 1500); counterAnim("#count3", -1000, -150, 2000); counterAnim("#count4", 500, -100, 2500); });
That’s all! hopefully, you have successfully integrated this animated website background code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
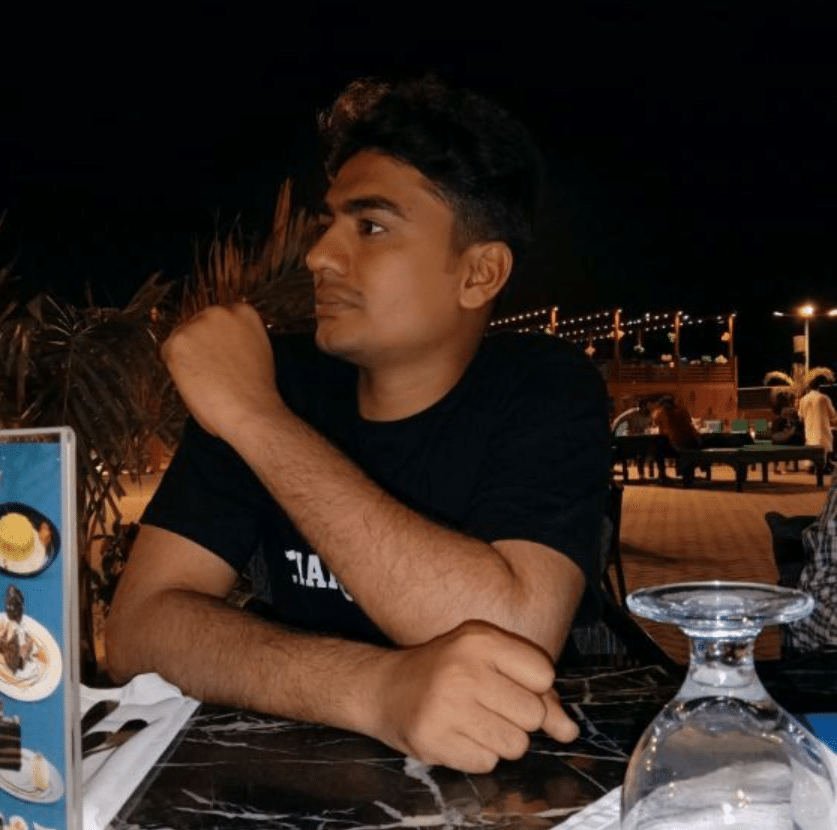
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.
Very beautiful, thank you!
Hi Uostau Pizdas!
You’re welcome! Keep visiting us for more free web design code and scripts.