The Counter JS is a lightweight JavaScript plugin to create an animated number counter. It uses the requestAnimationFrame() method to perform counting animation on given numbers. You need to pass the selector, starting & ending number, and duration of animation inside the function arguments and this plugin will animate the numbers.
How to Create Animated Number Counter JS
1. In order to create an animated number, you need to create a span element with a unique id. You can create multiple elements for counting animation on a single page. The following is the HTML structure:
<div class="container py-5 text-white"> <div class="row justify-content-center text-center"> <div class="col-12"> <h1 class="pb-3 h5">Number counter animation <br> in pure JavaScript <br> (requestAnimationFrame) </h1> </div> <div class="col-md-3"> <span id="count1" class="display-4"></span> </div> <div class="col-md-3"> <span id="count2" class="display-4"></span> </div> <div class="col-md-3"> <span id="count3" class="display-4"></span> </div> <div class="col-md-3"> <span id="count4" class="display-4"></span> </div> </div> </div>
2. After that, add the following CSS styles to your project:
/* I'm too lazy to write CSS 🌠... other than that Bootstrap has nothing to do with this example. */ body { background-color: #000; } main{ background: #8E2DE2; /* fallback for old browsers */ background: -webkit-linear-gradient(to right, #4A00E0, #8E2DE2); /* Chrome 10-25, Safari 5.1-6 */ background: linear-gradient(to right, #4A00E0, #8E2DE2); /* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */ }
3. Finally, add the following counter JS code before closing the body tag and done.
//#region - start of - number counter animation const counterAnim = (qSelector, start = 0, end, duration = 1000) => { const target = document.querySelector(qSelector); let startTimestamp = null; const step = (timestamp) => { if (!startTimestamp) startTimestamp = timestamp; const progress = Math.min((timestamp - startTimestamp) / duration, 1); target.innerText = Math.floor(progress * (end - start) + start); if (progress < 1) { window.requestAnimationFrame(step); } }; window.requestAnimationFrame(step); }; //#endregion - end of - number counter animation document.addEventListener("DOMContentLoaded", () => { counterAnim("#count1", 10, 300, 1000); counterAnim("#count2", 5000, 250, 1500); counterAnim("#count3", -1000, -150, 2000); counterAnim("#count4", 500, -100, 2500); });
That’s all! hopefully, you have successfully integrated this animated number counter code snippet into your project. If you have any questions or are facing any issues, please feel free to comment below.
Similar Code Snippets:
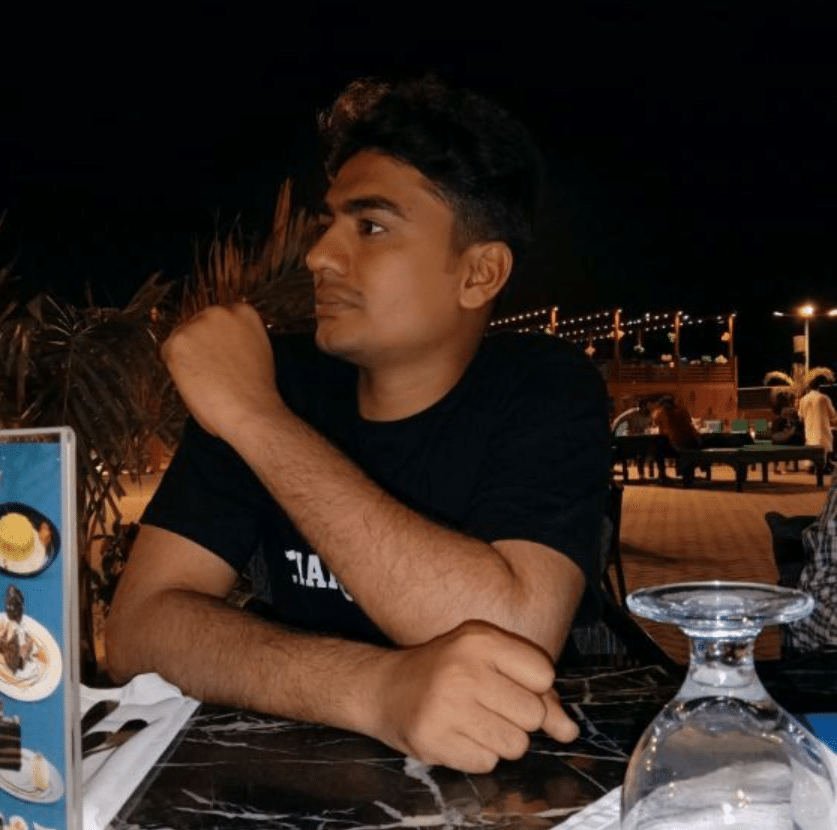
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.
thank you very much bro,i appreciate our help
Hi Tajir!
Thanks for your feedback. Keep visiting us for more free web design code and scripts.
Is there a way to modify the code to include commas for larger numbers?
THX very much. This works great.
When integrated in a website, how do I get animations to start counting at the moment they appear on display / when scrolled oder the section?