This Vanilla JavaScript code snippet helps you to create a simple image slider with dots navigation. It comes with fading transition effect while switching next and previous images.
How to Create Simple Image Slider in Vanilla JavaScript
1. First of all, create the HTML structure as follows:
<div class="container"> <div id="slider" class="slider"> <div class="slider-item active"><img src="https://loremflickr.com/1360/768/nature?random=1" alt="" class="img-fluid"></div> <div class="slider-item"><img src="https://loremflickr.com/1360/768/nature?random=2" alt="" class="img-fluid"></div> <div class="slider-item"><img src="https://loremflickr.com/1360/768/nature?random=3" alt="" class="img-fluid"></div> <ul id="dots" class="list-inline dots"></ul> </div> </div>
2. After that, add the following CSS styles to your project:
.slider .slider-item{ display: none; animation: reveal .5s ease-in-out; } .slider .slider-item.active { display: block; } .slider .dots { text-align: center; padding: 10px; } .slider .dots li { cursor: pointer; display: inline-block; background: #333; color: #fff; padding: 7px 11px; line-height: .5; border-radius: 50%; text-indent: -9999px; opacity: .5; -webkit-transition: opacity .2s; -o-transition: opacity .2s; transition: opacity .2s; } .slider .dots li.active{ opacity: 1; } @keyframes reveal{ from{ opacity: 0; } to{ opacity: 1; } }
3. Finally, add the following JavaScript code and done.
var slider = document.getElementById('slider') var sliderItem = slider.getElementsByTagName('div'); var dots = document.getElementById('dots'); var dotsChild = document.getElementById('dots').getElementsByTagName('li'); for (i = 0; i < sliderItem.length; i++) { dots.appendChild(document.createElement('li')); dotsChild[i].classList.add('list-inline-item'); dotsChild[i].setAttribute("id", i); dotsChild[i].innerHTML = i; dotsChild[0].classList.add('active'); dotsChild[i].addEventListener("click", runSlider); } function runSlider(){ var dnum = this.getAttribute("id"); for (i = 0; i < sliderItem.length; i++) { sliderItem[i].classList.remove('active'); sliderItem[dnum].classList.add('active'); dotsChild[i].classList.remove('active'); dotsChild[dnum].classList.add('active'); } }
That’s all! hopefully, you have successfully integrated this image sider code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
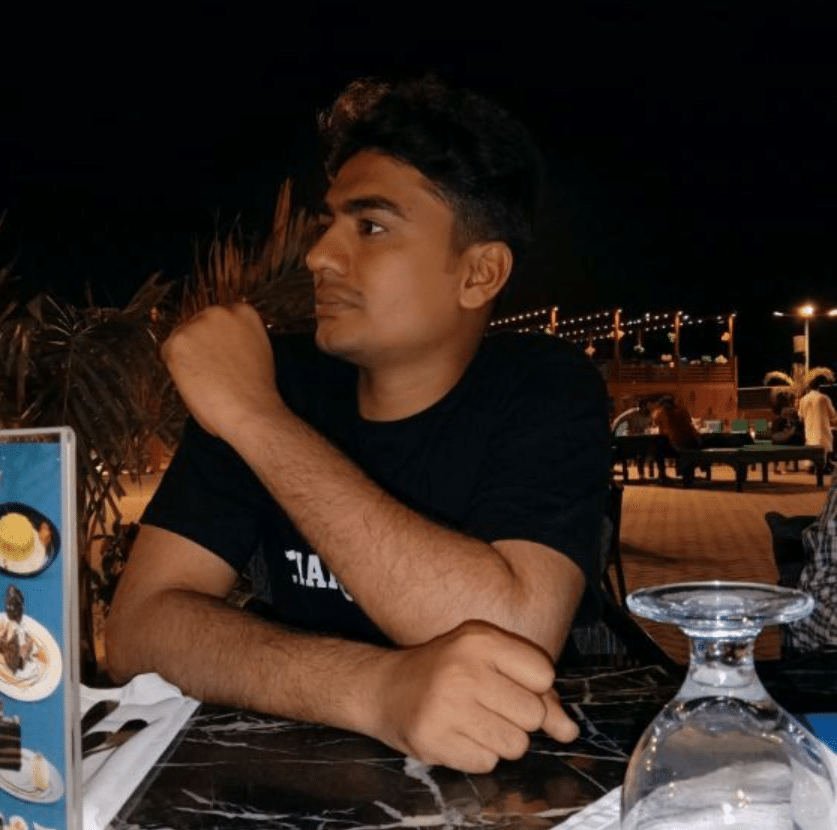
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.