This code implements a responsive stop watch with milliseconds using Bootstrap 5. The stopwatch displays hours, minutes, seconds, and milliseconds. You can start and stop the stopwatch with the “Start/Stop” button and reset it with the “Reset” button. The circular frame and controls are styled with Bootstrap, ensuring a visually appealing and user-friendly design. It’s a helpful tool for tracking time accurately in various scenarios.
How to Create Stop Watch With Milliseconds Using Bootstrap 5
1. First of all, include the necessary Bootstrap and Font Awesome CDN links in the <head>
section of your HTML file.
<!-- Bootstrap 5 CSS --> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"> <!-- Font Awesome Icons --> <link href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css" rel="stylesheet">
2. In HTML, create a container with the required elements for the stopwatch.
<div id="stopwatch-container"> <!-- Outer frame containing the circular frame and stopwatch --> <div id="outer-frame"> <div id="outer-circle"> <div id="stopwatch">00:00:00:00:00:00:000</div> </div> </div> <!-- Controls for the stopwatch --> <div id="controls"> <button class="btn btn-primary" onclick="startStop()"> <i class="fas fa-play"></i> Start/Stop </button> <button class="btn btn-danger" onclick="reset()"> <i class="fas fa-stop"></i> Reset </button> </div> </div>
3. Add the required styles to make the stopwatch visually appealing. Customize the colors, fonts, and sizes according to your preference.
body { font-family: 'Arial', sans-serif; display: flex; align-items: center; justify-content: center; height: 100vh; margin: 0; background-color: #2c3e50 !important; /* Dark grey background color */ color: #ecf0f1; /* Light text color */ } #stopwatch-container { text-align: center; } #outer-frame { width: 260px; height: 260px; border: 5px solid #ecf0f1; /* Circular frame color */ border-radius: 50%; display: flex; align-items: center; justify-content: center; margin: 0 auto; /* Center the frame */ } #outer-circle { width: 220px; height: 220px; border-radius: 50%; background-color: #3498db; display: flex; align-items: center; justify-content: center; } #stopwatch { font-size: 2em; color: #fff; } #controls { margin-top: 20px; } button { font-size: 1em; padding: 10px 20px; margin: 0 5px; cursor: pointer; }
4. Now, load the Bootstrap 5 JS, Popper JS, and jQuery by adding the following CDN links before closing the body tag:
<!-- Bootstrap 5 JS, Popper.js, and jQuery --> <script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script> <script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
5. Finally, include the following JavaScript code for the stopwatch functionality. This code handles starting, stopping, resetting, and updating the display with the elapsed time:
let startTime; let accumulatedTime = 0; let running = false; function startStop() { if (running) { running = false; } else { startTime = null; requestAnimationFrame(updateTime); running = true; } } function reset() { accumulatedTime = 0; updateDisplay(); running = false; } function updateTime(timestamp) { if (!startTime) { startTime = timestamp; } if (!running) return; accumulatedTime = timestamp - startTime; updateDisplay(); requestAnimationFrame(updateTime); } function updateDisplay() { const totalMilliseconds = Math.floor(accumulatedTime); const days = Math.floor(totalMilliseconds / (24 * 60 * 60 * 1000)); const hours = Math.floor((totalMilliseconds % (24 * 60 * 60 * 1000)) / (60 * 60 * 1000)); const minutes = Math.floor((totalMilliseconds % (60 * 60 * 1000)) / (60 * 1000)); const seconds = Math.floor((totalMilliseconds % (60 * 1000)) / 1000); const milliseconds = totalMilliseconds % 1000; const timeString = `${formatTime(days)}:${formatTime(hours)}:${formatTime(minutes)}:${formatTime(seconds)}:${formatTime(milliseconds, 3)}`; document.getElementById('stopwatch').innerText = timeString; } function formatTime(value, digits = 2) { const paddedValue = value.toString().padStart(digits, '0'); return paddedValue.substring(0, digits); } // Add event listener to stop the stopwatch on any touch $(document).on('touchstart', function () { running = false; }); // Initial display updateDisplay();
That’s all! hopefully, you have successfully created a stopwatch with milliseconds using Bootstrap 5. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
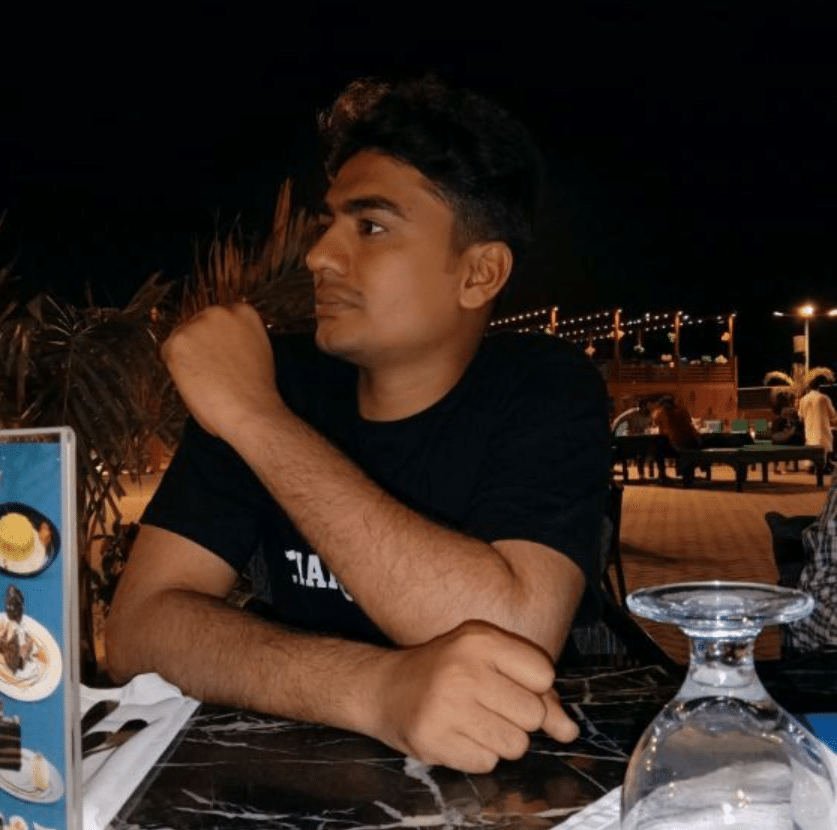
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.